While loops are powerful, Python offers a concise way to create lists using list comprehension. Imagine you need a list containing squares of numbers from 1 to 5. Here’s how to do it with a loop:
A Pythonic Shortcut for List Creation
squares = []
for i in range(1, 6):
squares.append(i * i)
print(squares) #This will Output: [1, 4, 9, 16, 25]
With just one line of code, you can create the same list using a list comprehension. The for loop and the addition of additional elements are combined into a single line in a list comprehension, which automatically adds every additional ingredient. We can rewrite the above code of creating a list with items of squares.
squares = [value**2 for value in range(1, 6)]
print(squares)
Now let count, one to twenty using list comprehension. We will use a for loop to print the numbers from 1 to 20, inclusive.
#we begin by creating the list
numbers = [number for number in range(1,21)]
print(numbers)

Let’s count to one million once more using this example: Create a list of all the numbers between one and one million, and then print the numbers using a for loop. (If the output is taking too long, you can end it by closing the output window or by pressing Ctrl-C.)
oneToOneMillion = [number for number in range(1, 1000001)] #create the list
print(oneToOneMillion) #print the list

IF you look at the result in the VSCode terminal, you can see that the numbers counted couldn’t be printed up to one million. In fact, it stopped after 2752. I think this was the VSCode problem. It did try, though.
Now, let us do Summing a Million: Make a list of the numbers from one to one million, and then use min() and max() to make sure your list actually starts at one and ends at one million. Also, use the sum() function to see how quickly Python can add a million numbers.
oneToOneMillion = [number for number in range(1, 1000001)] #create the list
print(min(oneToOneMillion))
print(max(oneToOneMillion))
print(sum(oneToOneMillion))
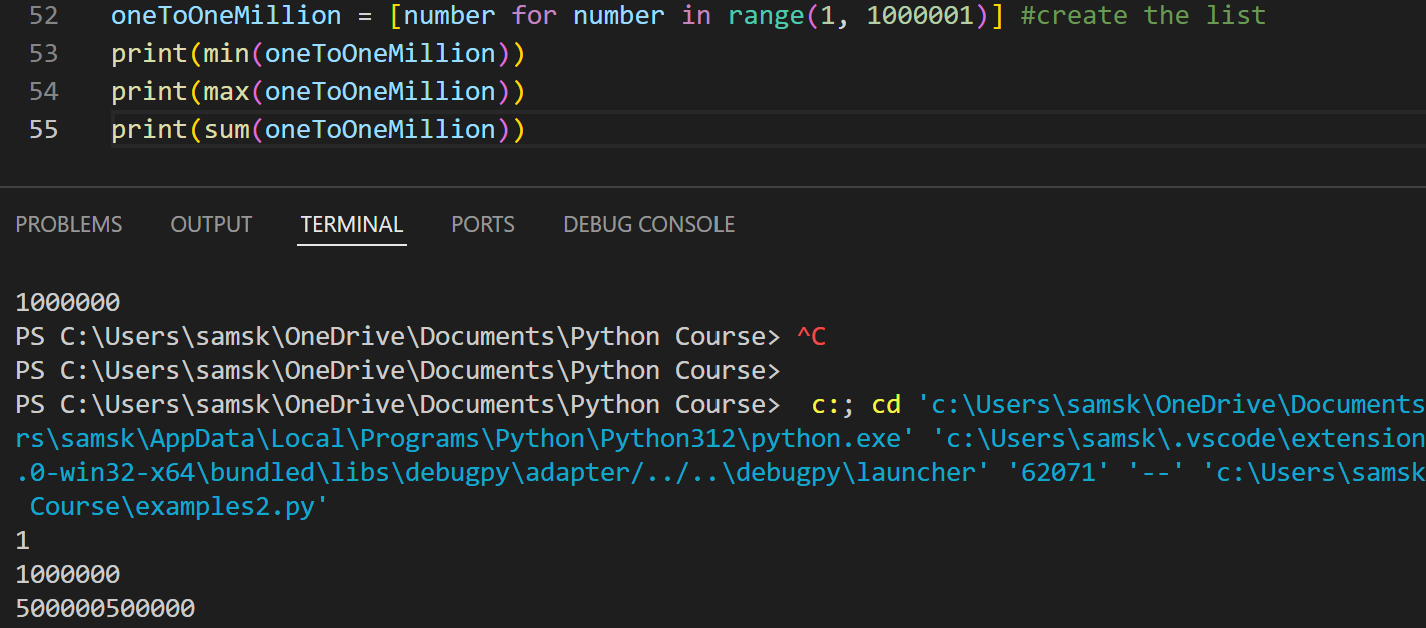
Exercises on Lists and List Comprehension
- Odd Numbers: To create a list of the odd numbers between 1 and 20, use the range() function’s third argument. For each number, use a for loop to print it.
- Threes: Enumerate the multiples of three between three and thirty. To print the numbers in your list, use a for loop.
- Cubes: A cube is a number raised to the third power. For instance, in Python, the cube of 2 is represented as 2**3. Using a for loop, generate a list of the first 10 cubes, or the cube of each number from 1 to 10, and print the value of each cube.
- Cube Comprehension: Make a list of the first ten cubes using a list comprehension technique.
Read More Posts Like This…
- Working With Lists In Python
- Introduction to Variables and Data Types in Python
- Women Who are Self-Made Billionaires: Top 15 In The World
- Unrecognized Threats to Mental Wellbeing
Copying a Lists in Python Programming
Copying an Entire List
originalList = ['lagos', 'kano', 'kaduna', 'lokoja', 'abuja'] #this is the original list of states in nigeria
copyList = originalList[:] #create an empty list without modifying the index
copyList = originalList #use this syntax to make the copy
print(originalList)
print(f"The copied list: \n{copyList}")
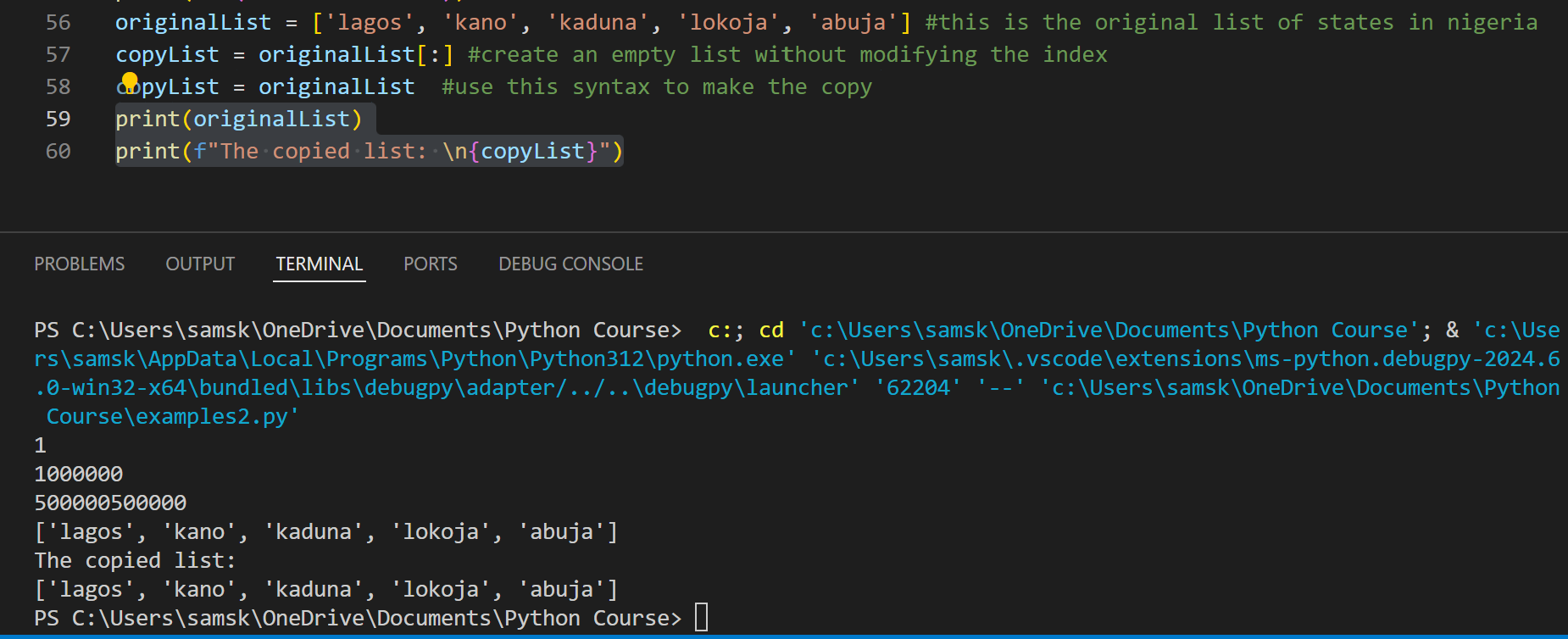
Nesting Lists: Building Lists Within Lists
party_games = [
["Board Games", "Alice", "Bob"],
["Video Games", "David", "Emily"]
]
This code creates a nested list named party_games
. The outer list contains two inner lists, each representing a game and its participants.
Example 1: Basic Nested List
# Creating a nested list
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Accessing elements in a nested list
print(nested_list[0]) # Output: [1, 2, 3]
print(nested_list[1][2]) # Output: 6
# Modifying an element in a nested list
nested_list[2][1] = 88
print(nested_list) #This should Output: [[1, 2, 3], [4, 5, 6], [7, 88, 9]]
A nested list is simply a list that contains other lists as its elements.
Example 2: Iterating Over a Nested List
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Using nested loops to iterate over the elements
for sublist in nested_list:
for item in sublist:
print(item, end=' ')
#this shoul output the following : 1 2 3 4 5 6 7 8 9
You can use nested loops to iterate over each element in a nested list.
Example 3: List Comprehension with Nested Lists
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Flattening a nested list using list comprehension
flattened_list = [item for sublist in nested_list for item in sublist]
print(flattened_list) #this will output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
List comprehension can also be used with nested lists.
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Using the `len` function to find the length of a nested list
print(len(nested_list)) # Output: 3
print(len(nested_list[0])) # Output: 3
# Using the `sum` function to sum elements of a sublist
print(sum(nested_list[1])) # Output: 15
# Creating a 3x3 matrix filled with zeros
matrix = [[0 for _ in range(3)] for _ in range(3)]
print(matrix)
# Output: [[0, 0, 0], [0, 0, 0], [0, 0, 0]]
# Filling the matrix with incrementing numbers
count = 1
for i in range(3):
for j in range(3):
matrix[i][j] = count
count += 1
print(matrix)
# Output: [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Transposing the nested list
transposed_list = [[nested_list[j][i] for j in range(len(nested_list))] for i in range(len(nested_list[0]))]
print(transposed_list)
# Output: [[1, 4, 7], [2, 5, 8], [3, 6, 9]]
More Practice Questions on List Comprehension
20 Questions to Test Your List Mastery
- Create a list containing the names of your favorite fruits.
- Print the number of elements in your fruit list.
- Add a new fruit to the end of your fruit list using the
.append()
method. - Access the second element in your fruit list and print it.
- Write a loop that iterates through your fruit list and prints each fruit along with its index position.
- Remove the first element from your fruit list using the
.pop(0)
method. - Check if a specific fruit is present in your list using the
in
operator. 8. Create a new list by slicing a portion of your fruit list. - Use list comprehension to create a list that squares all the numbers from 1 to 10.
- Create a nested list containing information about your favorite books (title, author, genre).
- Copy your fruit list using list slicing and compare it with the original list.
- Write a function that takes a list of numbers and returns the sum of all the elements.
- Extend your fruit list by adding another list containing additional fruits.
- Remove all occurrences of a specific fruit from your list using a loop.
- Sort your fruit list alphabetically using the
.sort()
method. - Reverse the order of your fruit list using the
.reverse()
method. - Concatenate your fruit list with another list containing your favorite vegetables to create a combined list.
FAQs on List Comprehension
What are some real-world applications of using lists in Python?
Python lists have a wide range of applications. Here are a few examples:
- Storing and processing sensor data: Lists can be used to store and analyze data collected from sensors in various applications like robotics or Internet of Things (IoT) projects.
- Developing interactive games: Lists can be used to manage game elements like character stats, inventory items, or high score lists.
- Data analysis and machine learning: Lists are essential for storing and manipulating datasets used in data analysis and machine learning algorithms.
- Web scraping and data extraction: Lists can be used to store and organize data extracted from websites during web scraping tasks.
2. When should I use lists versus other data structures in Python?
Lists are a good choice for ordered collections of elements that can change dynamically. However, if you need more specific functionalities, consider other data structures:
- Tuples: Use tuples for immutable ordered collections where elements cannot be modified after creation.
- Sets: Use sets for unordered collections of unique elements, helpful for checking element membership or performing set operations.
- Dictionaries: Use dictionaries for key-value pairs, allowing you to associate data with unique keys for efficient retrieval.
3. How can I improve the efficiency of my code when working with large lists?
For very large lists, consider these optimization techniques:
- List comprehensions: They can be more memory-efficient than traditional loops for creating new lists.
- Generators: Generators create elements on-demand, reducing memory usage for large datasets compared to storing everything in a list at once.
- Built-in list methods: Utilize built-in methods like
.sort()
or.reverse()
for efficient list manipulation tasks.
4. What are some advanced list functionalities I can explore?
As you progress in Python, explore these advanced list concepts:
- List unpacking: Unpack elements from a list into variables for concise code.
- Generators expressions: Create generators for memory-efficient iteration.
- Lambda functions: Use lambda functions with list methods for compact anonymous functions within list operations.
5. Where can I find more resources to learn about working with lists in Python?
The official Python documentation is a great starting point, check out the Python website for this here. Additionally, online tutorials, forums, and books dedicated to Python programming can provide further in-depth explanations and practice exercises.