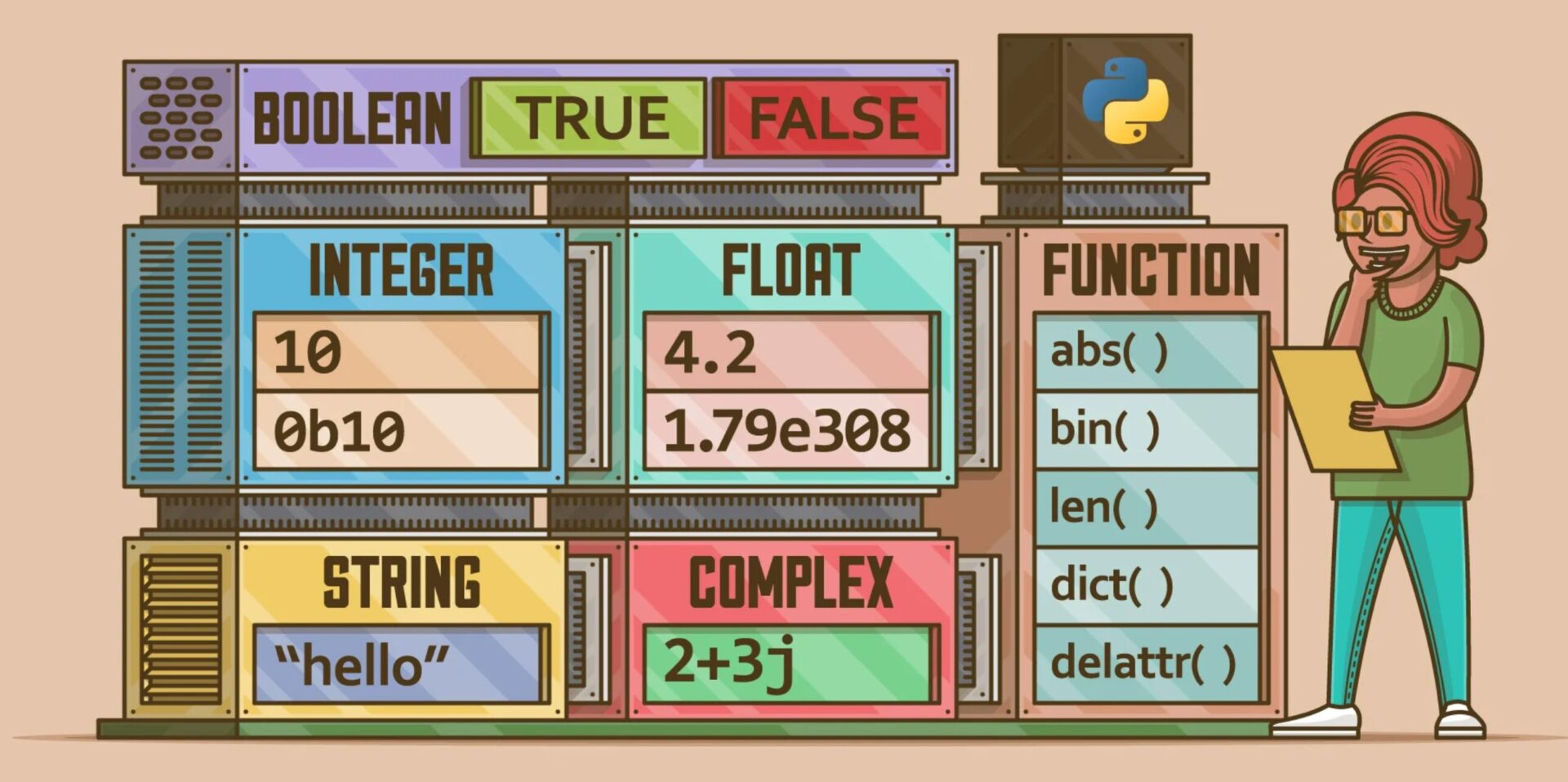
When working with variables in Python, it is important to understand the concept of variables and data types. A data type is a classification of data that determines the operations that can be performed on it and the values that it can take. Python has several built-in data types, including:
- Integer (int): This data type represents whole numbers, both positive and negative. For example, 5 and -10 are integers.
- Float (float): This data type represents decimal numbers. For example, 3.14 and -2.5 are floats.
- String (str): This data type represents a sequence of characters. Strings are enclosed in single or double quotes. For example, ‘hello’ and “world” are strings.
- Boolean (bool): This data type represents a binary value, either True or False. Booleans are used for logical operations and comparisons.
- List (list): This data type represents an ordered collection of items. Lists can contain elements of different data types and are enclosed in square brackets. For example, [1, ‘apple’, True] is a list.
- Tuple (tuple): This data type is similar to a list, but it is immutable, meaning that its elements cannot be changed once assigned. Tuples are enclosed in parentheses. For example, (1, ‘apple’, True) is a tuple.
- Dictionary (dict): This data type represents a collection of key-value pairs. Dictionaries are enclosed in curly braces and consist of keys and their corresponding values. For example, {‘name’: ‘John’, ‘age’: 25} is a dictionary.
Understanding and correctly using these data types is crucial for writing efficient and effective Python code. By choosing the appropriate data type for a variable, you can optimize memory usage and ensure that operations are performed correctly. Python also allows for type conversion, which enables you to convert a variable from one data type to another.
In addition to the built-in data types, Python also provides the ability to define custom data types using classes. Classes allow you to create objects that have their own attributes and methods, providing a way to organize and manipulate complex data.
Overall, variables and data types are fundamental concepts in Python programming. By mastering them, you will have a solid foundation for writing powerful and efficient code.
What Do You understand As a Variable?
Some people regard variables as boxes you can save or put your data type in. This idea could only work for you for some times but it isn’t a complete accurate way to describe how variables are represented in Python Programming language. Variables are labels that you assign to a value. This means that a variable reference a particular value.
Common Data Types in Python
Python provides several built-in data types that can be used to store different kinds of values. Here are some of the most commonly used data types in Python:
1. Numeric Data Types
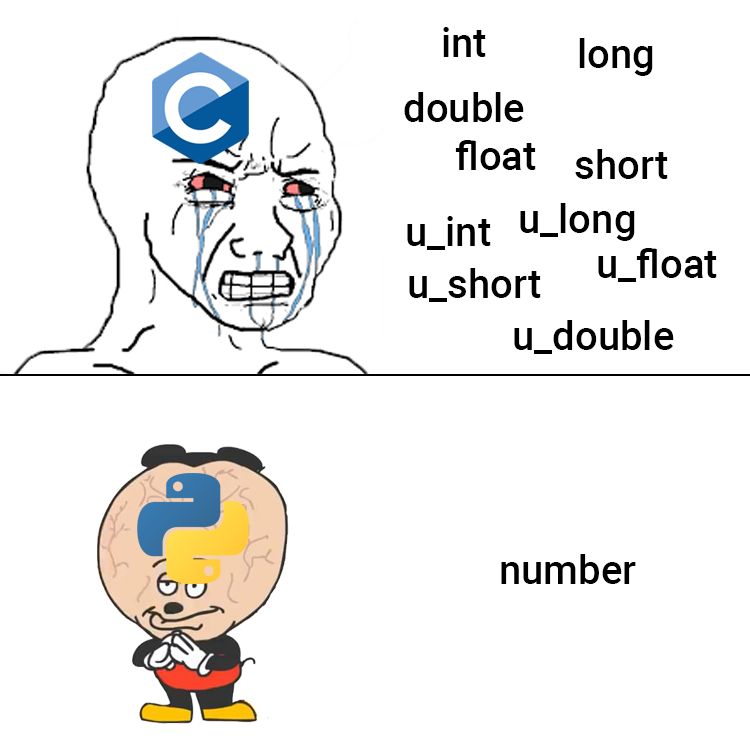
Python supports various numeric data types, including:
- Integer (int): This data type is used to represent whole numbers, both positive and negative.
- Float: This data type is used to represent decimal numbers.
- Complex: This data type is used to represent complex numbers in the form a + bj, where a and b are floats and j is the imaginary unit.
Here are some examples of variables using numeric data types:
num1 = 10
num2 = 3.14
num3 = 2 + 3j
More on this later in Numbers and Operation…
2. String Data Type
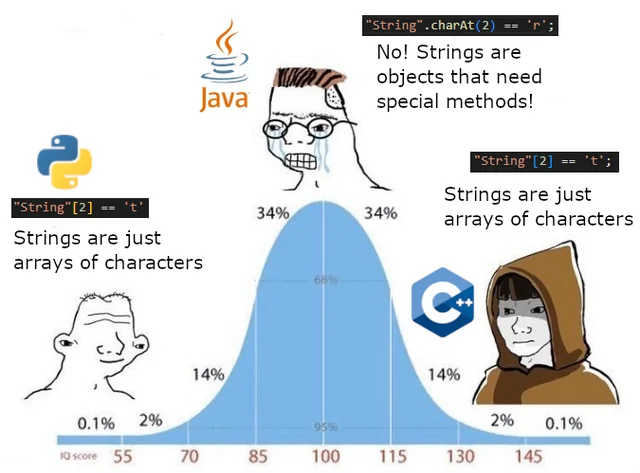
The string data type is used to represent a sequence of characters. Strings are enclosed in either single quotes (”) or double quotes (“”).
Here is an example of a variable using the string data type:
name = "John Doe"
We will dive deeper below soonest.
3. Boolean Data Type
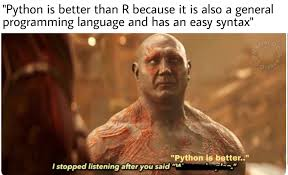
The Boolean data type is used to represent the truth values True and False. It is often used in conditional statements and logical operations.
Here are some examples of variables using the Boolean data type:
is_active = True is_admin = False
4. List Data Type
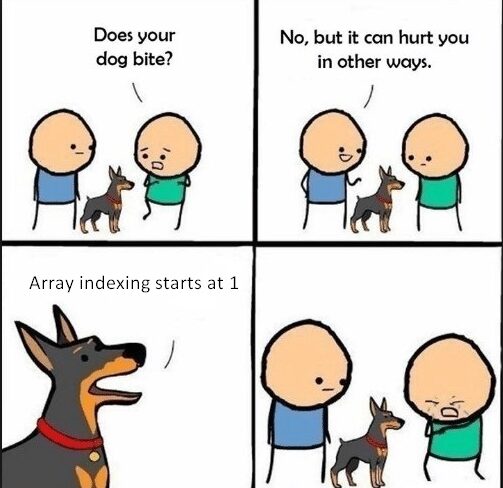
The list data type is used to represent an ordered collection of items. Lists can contain elements of different data types and are mutable, meaning that their elements can be modified.
Here is an example of a variable using the list data type:
fruits = ["apple", "banana", "orange"]
Read More Posts Like This…
- How The Internet Works
- How Do Websites Work: Basics of Front-End Web Development
- Unaware Alcohol, Linked to Breast Cancer In Women – WHO
- 7 Breakfast Foods You Need for Your Body Every Morning
5. Tuple Data Type
The tuple data type is similar to a list, but it is immutable, meaning that its elements cannot be modified once it is created. Tuples are often used to represent a collection of related values.
Here is an example of a variable using the tuple data type:
coordinates = (10, 20)
6. Dictionary Data Type
The dictionary data type is used to represent key-value pairs. Each element in a dictionary is a pair consisting of a key and its corresponding value. Dictionaries are mutable and can be modified.
Here is an example of a variable using the dictionary data type:
person = {"name": "John Doe", "age": 30, "city": "New York"}
Understanding these common data types in Python is essential for writing effective and efficient code. By using the appropriate data type for each value, you can ensure that your programs are accurate and performant.
In addition to these built-in data types, Python also allows you to define your own custom data types using classes and objects. This object-oriented approach provides flexibility and allows you to create data structures tailored to your specific needs.
Overall, Python’s rich set of data types makes it a versatile and powerful programming language for a wide range of applications.
Rules for Naming Variables
When naming variables in Python, there are some rules that need to be followed:
- A variable name must start with a letter or an underscore (_).
- A variable name can only contain letters, numbers, and underscores.
- A variable name cannot be a reserved word or keyword.
- Variable names are case-sensitive.
- Variable names should be meaningful and descriptive.
Choosing appropriate variable names is an important aspect of writing clean and readable code. Descriptive variable names make it easier for other programmers (and even yourself) to understand the purpose or meaning of the data they represent. This can greatly enhance the maintainability and readability of your code.
For example, instead of using a generic variable name like “x” or “temp”, it is better to use more descriptive names like “num_of_students” or “average_temperature”. This makes it clear what the variable represents and improves the overall clarity of your code.
In addition to choosing descriptive names, it is also important to follow consistent naming conventions. This helps maintain a standard and uniform style throughout your codebase. Some common conventions include using lowercase letters for variable names and separating words with underscores (snake_case).
By following these rules and best practices for naming variables, you can improve the readability, maintainability, and overall quality of your Python code.
Examples of These Rules in Python
- Starting a variable like name_1 is allowed and correct, but not the variable 1_name.
- Beware of the lowercase letter “l” and upper case “O” as they can be confused for the number 1 and 0 when programming in Python.
- Avoid the use of abbriviations, use the variable “other_names” instead of “o_n”.
- Lastly, try as much as you can to stick to using lowercase characters for variable names. You won’t get an error if you use upper case letters but in python programming language that is reserved for a special case.
Practice Questions
Now that you have learned about variables and data types in Python, here are some practice questions to test your understanding:
- Create a variable called “age” and assign it the value 25.
- Create a variable called “name” and assign it your name as a string.
- Create a list called “numbers” and add the numbers 1, 2, and 3 to it.
- Create a dictionary called “person” with the keys “name”, “age”, and “city”, and assign them appropriate values.
- Perform arithmetic operations on the variables “num1” and “num2” and store the results in a new variable called “result”.
- Create a variable called “is_even” and assign it the value of checking whether the number stored in “result” is even or not.
- Print the value of “is_even” to the console.
- Create a variable called “is_divisible_by_3” and assign it the value of checking whether the number stored in “result” is divisible by 3 or not.
- Print the value of “is_divisible_by_3” to the console.
Take your time to solve these questions and feel free to refer back to the examples and explanations provided above. Practice is key to mastering variables and data types in Python programming language.
Deeper Dive In String Data Type in Python
As mentioned earlier, a string in Python programming language is a series of characters. Unlike the char data type in C/C++ language, anything inside a quote is considered a String. Examples:
- ‘My name is Anc.’
- “The name ‘Python’ programming language came from the British Comic/Band Monty Python not the snake”
- “One of Python’s strength is its diverse community”
Changing Case In String with Methods
Open your VSCode editor and create a new Python file. For me, I named it strings.py. You can start practicing your string variable examples here.
name = "emmanuel kant"
print(name.title())
Examine the subsequent code above and try running it on your editor. Then, attempt to ascertain what is happening there.
name = "Ada Lovelace"
print(name.upper())
print(name.lower())
first_name = "emmanuel"
last_name = "kant"
full_name = f"{first_name} {last_name}"
print(full_name)
In the above code we used the f-strings variable and it is very useful when printing strings.
This is also a form of string concatenation which means joining strings together.
print(f"Hello, {full_name.title()}!")
The f is for format, because Python formats the string by replacing the name of any variable in braces with its value.
Manipulating String Data Type
#create a string variable named message
checkList = "Not bulging"
message = 'hello world python crash course'
#print out the message in terminal on a new line
print(f"{checkList}'\n' {message}")
#just print out hello world
print("hello world")
#print more string messages
message = 'I told my friend that the "programming language python" came from the band "Monty Python" '
print(message)
#create a variable name
name = 'ada lovelace'
#print the variable in upper case
print(name.upper())
#begin each word with capital letter
print(name.title())
firstName = 'eureka'
lastName = 'nwachukwu'
#use the f string in python
fullName = f'{firstName} {lastName}'
print(fullName.title())
#use also the f-string to add another string message in print
print(f"hello {fullName.title()}")
greeting = f'hello Miss E'
#use the strip() to remove whitespaces
print(greeting.rstrip())
Adding and Removing Whitespace and Solving Name Error In Python

Whitespace can help you arrange your content so that readers can read it more easily. In programming, whitespace refers to any nonprinting character, such as spaces, tabs, and end-of-line symbols.
You can use the the method strip() to remove the whitespaces on both side of a string. You can also specify by using the left strip like rstrip() to remove the right side of the whitespace or lstrip() to remove the left side of the whitespace.
print("Here are the four best programmign languages: \n\tPython\n\tC++\n\tJavaScript\n\tPHP")
To add whitespace use, tabs and new lines syntax as shown above.
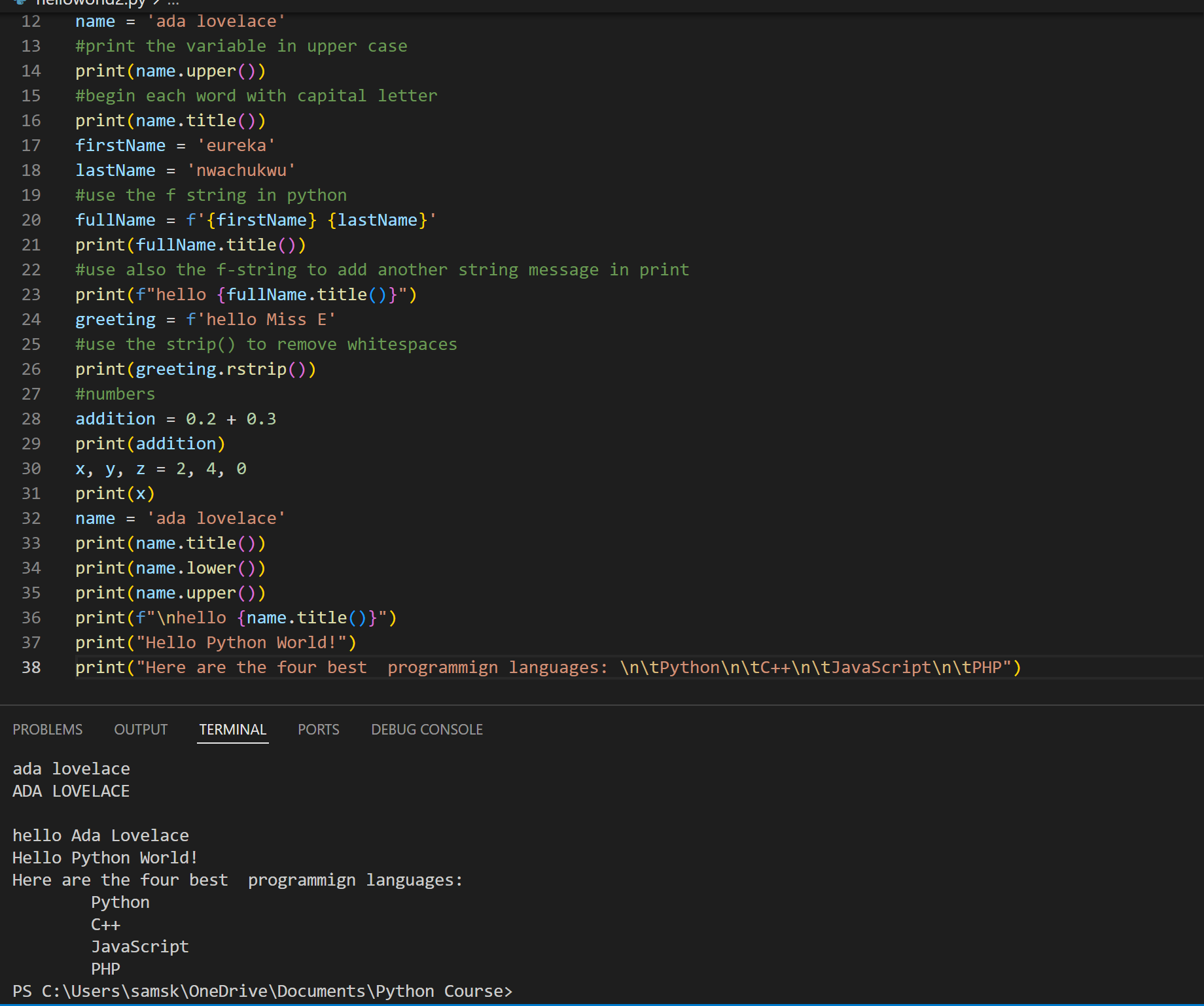
When you are programming and you get an error, when you get a name error type, follow the traceback on your console or terminal . The interpreter helps you identify which line of code with the error and tells you what type of error it is. And in most cases with strings, it is a a name type error.
Conclusion
Having discussed in full detail what variables are in Python programming language and the various data types, we would like to know your thoughts. Practice the following quiz below.
Practice Questions
- What is the output of the following code?
my_string = "Hello, World!"
print(my_string[7])
A) ‘W’
B) ‘o’
C) ‘r’
D) ‘d’
2. Which of the following methods can be used to convert a string to uppercase?
A) upper()
B) toUpperCase()
C) uppercase()
D) upperCase()