Python is a versatile and powerful programming language that is widely used in various fields, including data science, machine learning, web development, and scientific computing. One of the key features of Python is its ability to handle numbers and perform various operations on them efficiently. In this guide, we will explore the fundamentals of numbers and operations in Python programming, from basic arithmetic to more advanced concepts
Numbers and Operations: The Bedrock of Python Programming
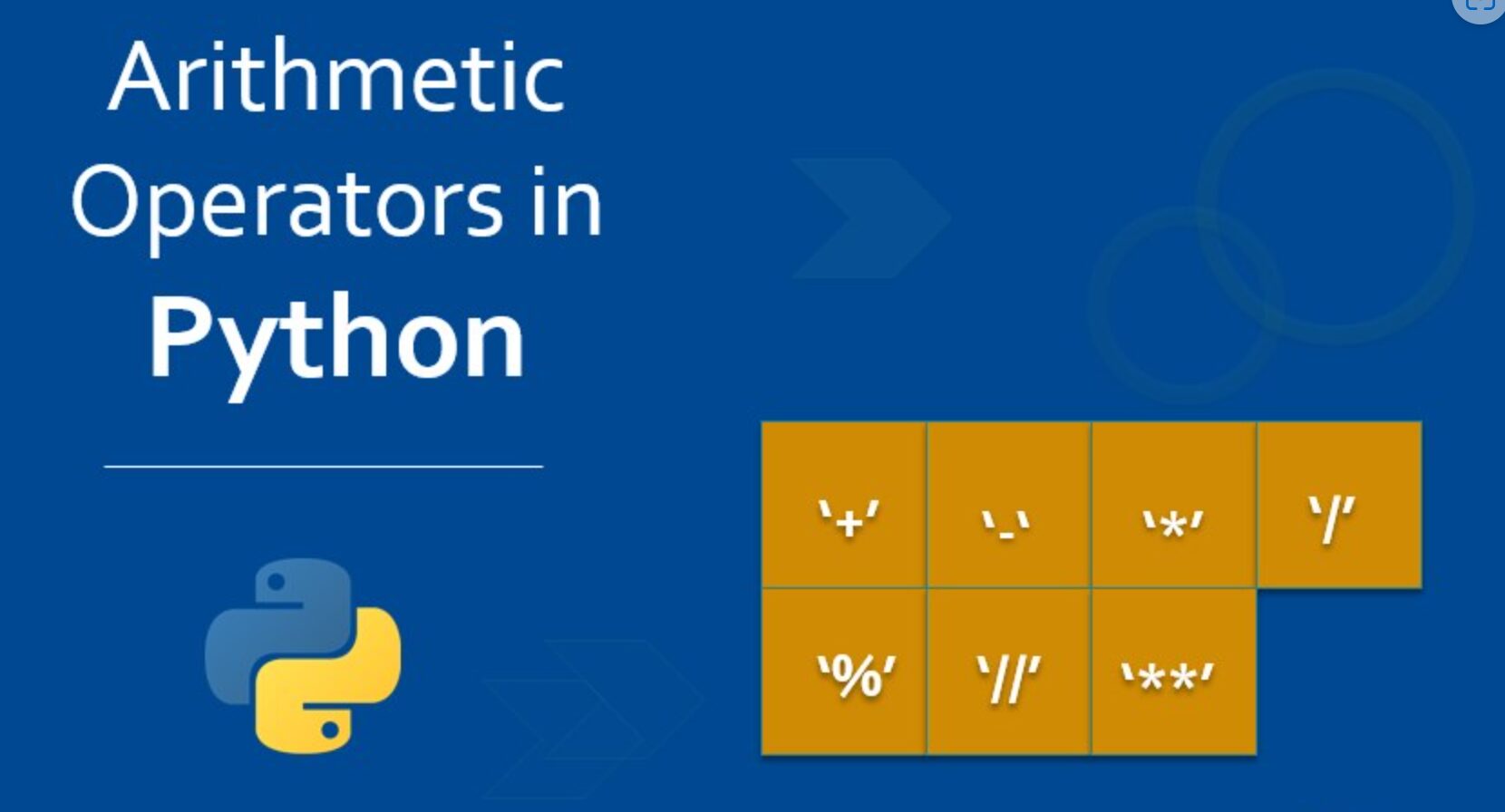
Welcome to the thrilling world of Python programming, where numbers and operations are the building blocks that bring your code to life! Whether you’re a seasoned coder or a curious beginner, this guide will equip you with the fundamental knowledge to manipulate numbers and perform calculations with Python’s intuitive syntax. Buckle up, and let’s dive into the fascinating realm of numeric data types and operations!
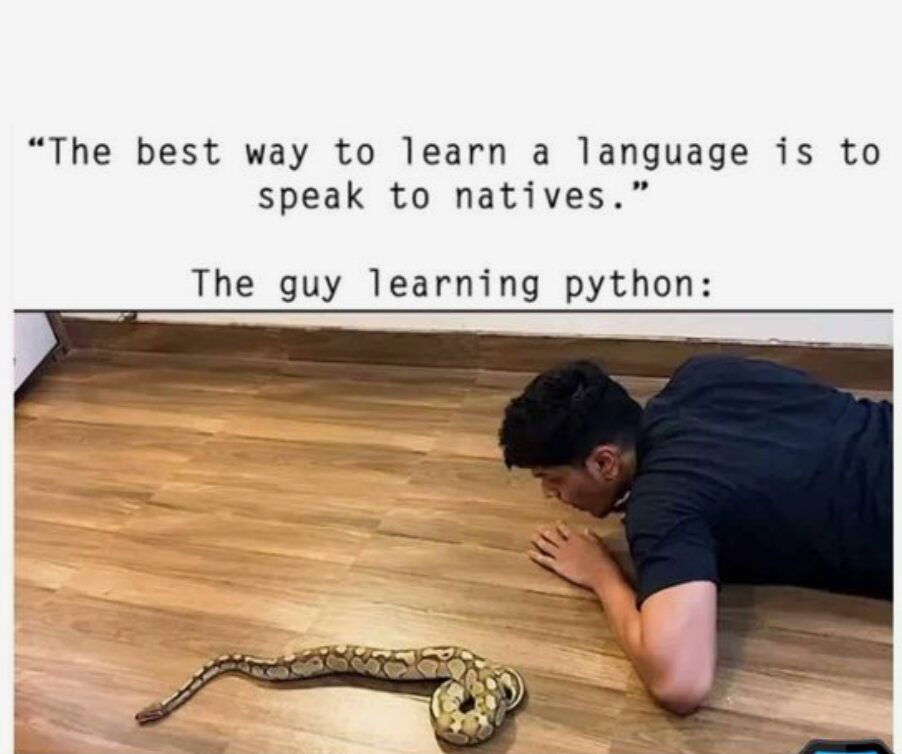
it’s important to understand the basic data types in Python. Python supports several types of numbers, including integers, floating-point numbers, and complex numbers. Integers are whole numbers without any decimal point, floating-point numbers are numbers with a decimal point or an exponential form, and complex numbers are numbers with a real and imaginary part.
The Numeric Kingdom: A Tour of Python’s Data Types
Python, unlike some uptight rulers, embraces a variety of numeric citizens. Here’s a peek into the most common ones:
- Integers (int): These are the whole number workhorses, like counting apples (1, 2, 3) or calculating years (2023).
- Floats (float): Imagine numbers with decimal points, like representing pi (3.14159) or measuring distances (10.5 kilometers).
- Complex numbers (complex): These combine the real world (integers and floats) with the imaginary unit (i), venturing into the realm of advanced mathematics.
Assigning Values
Imagine having a box full of Legos, but none are labeled! Thankfully, Python allows us to assign names (variables) to our numbers for easy reference. Here’s how:
age = 30
pi = 3.14159
Now, you can use age
and pi
throughout your code without memorizing the actual values. Also, you can:
a = 5
b = 3
sum = a + b
print(sum)
Performing Calculations: The Power of Python’s Operators
Python offers a rich arsenal of operators to perform various calculations on numbers. Let’s explore some key ones:
- *Arithmetic Operators (+, -, , /, //, %): These are the familiar math symbols you know and love, allowing you to add, subtract, multiply, divide (including integer division with // and modulo with %).
- Comparison Operators (==, !=, <, >, <=, >=): These compare numbers and return True or False. Imagine judging if a number is equal to another (==) or greater than (>).
- *Assignment Operators (=, +=, -=, =, /=, //=): These not only assign values but also perform calculations. For example,
x += 5
is shorthand forx = x + 5
.
a = 3 + 4j
b = complex(5, 6)
print(a * b)
Mastering Expressions: Combining Operators and Values
Think of an expression as a mini-formula. You can combine variables, numbers, and operators to create meaningful calculations. Here’s an example:
total_cost = price * quantity + shipping_fee
This expression calculates the total cost by multiplying price and quantity, then adding the shipping fee.
Operations With Floating Point Numbers
Try the following code in your Visual Studio Editor:
first_float = 0.1
second_float = 0.1
addition_of_float = first_float + second_float
print(addition_of_float)
third_float = 0.2
addition_of_float = first_float + third_float
print(addition_of_float)
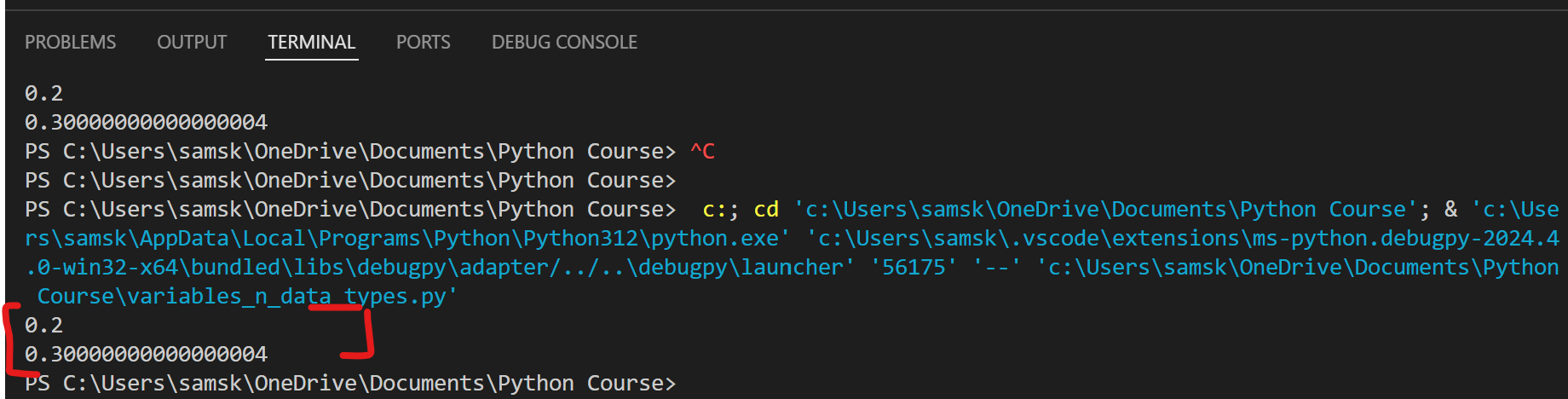
Notice the difference here. The first addition was pretty straight-forward and the other was having a little extra decimal places. This happens in all languages and is of little concern. Python tries to find a way to represent the result as precisely as possible, which is sometimes difficult given how computers have to represent numbers internally. Just ignore the extra decimal places for now.
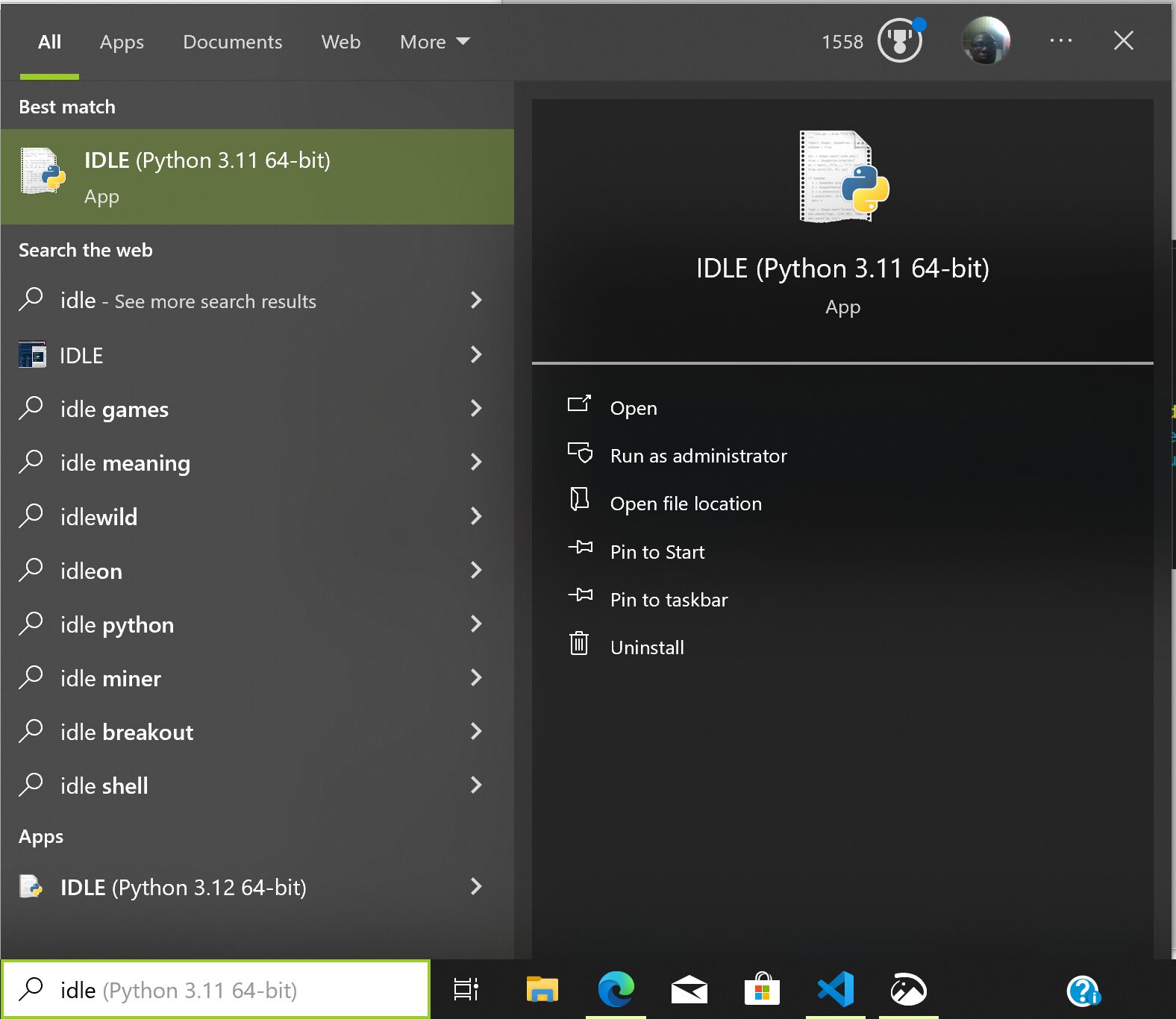
Type “IDLE” in the search of your Windows, and you can open the default Python Editor. Try reproducing the following below.
>>> 4/2
2.0
>>> 1 + 2.0
3.0
>>> 2 * 3.0
6.0
>>> 3.0 ** 2
9.0
When you divide any two numbers, even if they are integers that result in a whole number, you’ll always get a float. If you mix an integer and a float in any other operation, you’ll get a float as well. This is because Python defaults to a float in any operation that uses a float, even if the output is a whole number.
Numbers and Operations in Python: Underscores In numbers
dinosaur_extinction_age = 2_000_000
print(dinosaur_extinction_age)
To make long numbers easier to read while writing, you can use underscores to group digits together. as shown above. Python outputs just the digits when you print a number that was declared using underscores. When saving these kinds of values, Python ignores the underscores. The value won’t change whether or not you group the digits in threes. 1000 is equivalent to 1_000, which is equivalent to 10_00 in Python. This feature is limited to Python 3.6 and higher versions, and it functions for both integers and floats.
The Zen of Python
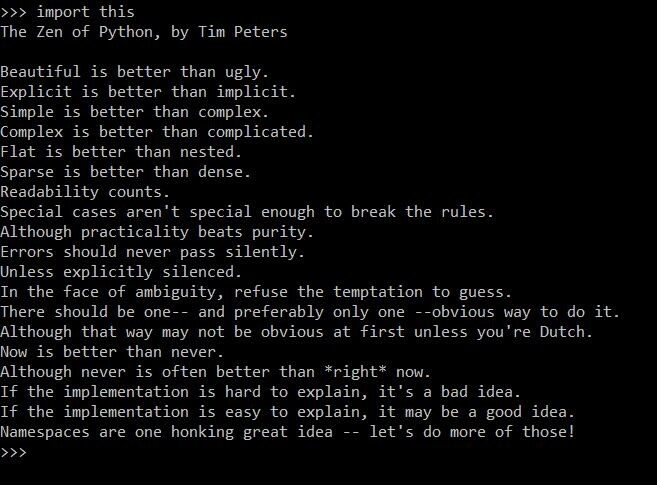
“The Zen of Python” is a collection of aphorisms that capture the guiding principles behind Python’s design and philosophy. These aphorisms, also known as PEP 20 (Python Enhancement Proposal 20), were written by Tim Peters and serve as a set of guidelines for Python developers to follow when writing code. In this outline, we will discuss the key themes and principles of “The Zen of Python” and how they influence Python development.
Every skilled Python programmers would advise you to strive for simplicity and steer clear of complexity wherever you can. Tim Peters’ “The Zen of Python” contains the philosophy of the Python community. To obtain this concise collection of guidelines for crafting quality Python code, simply type “import this” into your interpreter.
More Advanced Uses of Numbers and Operations In Python
Logical Operators (and, or, not)
Logical operators help us make decisions based on conditions. Imagine having a bouncer at a club (the code) who only lets people in if they meet certain criteria (conditions). Here’s how these operators work:
and
: Both conditions must be True for the overall expression to be True (like needing an ID and being over 21 to enter the club).or
: At least one condition must be True for the expression to be True (like having a VIP pass or being a friend of the owner).not
: Inverts the truth value of a condition (like checking if someone is not under 18).
Conditional Statements: Making Choices with if, elif, else
Conditional statements allow your code to take different paths based on whether a condition is True or False. Imagine a choose-your-own-adventure story, where the narrative unfolds based on your choices:
if age >= 18:
print("You are eligible to vote!")
elif age >= 16:
print("You can get a driver's permit!")
else:
print("Enjoy being a kid while it lasts!")
This code checks the age and prints different messages depending on the outcome.
The Power of Repetition (for, while)
- while loop: This keeps looping as long as a condition remains True. Imagine a hamster on a wheel, running as long as the wheel keeps spinning (the condition).
Here’s an example of a while loop
guessing a random number:
import random
secret_number = random.randint(1, 100)
guess = 0
while guess != secret_number:
guess = int(input("Guess a number between 1 and 100: "))
if guess > secret_number:
print("Too high! Try again.")
elif guess < secret_number:
print("Too low! Try again.")
else:
print("You guessed it! The secret number was", secret_number)
Built-in Functions for Efficiency: Built-in Mathematical Functions
Python provides a treasure trove of built-in functions for common mathematical operations, saving you time and effort. Here are a few examples:
abs(x)
: Returns the absolute value of a number (distance from zero).round(x, n)
: Rounds a number to n decimal places.pow(x, y)
: Calculates x raised to the power of y.max(x, y, ...)
: Returns the largest number from a sequence.min(x, y, ...)
: Returns the smallest number from a sequence.
These functions make your code more concise and readable.
Putting It All Together: Examples that Bring Concepts to Life
Now that you’ve explored the fundamentals, let’s see how these concepts work together in practical examples:
- Calculating the area of a rectangle:
length = 10
width = 5
area = length * width
print("The area of the rectangle is", area)
- Converting temperature from Celsius to Fahrenheit:
celsius = 25
fahrenheit = (celsius * 9/5) + 32
print("The temperature in Fahrenheit is", fahrenheit)
Error Handling: Gracefully Facing Challenges
Even the most seasoned programmers encounter errors. Python provides mechanisms to handle these gracefully, preventing your code from crashing. Here’s a basic example:
try:
age = int(input("Enter your age: "))
if age < 0:
raise ValueError("Age cannot be negative!")
print("Your age is", age)
except ValueError as e:
print("Error:", e)
This code attempts to convert user input to an integer but raises a ValueError
if the input is negative, providing an informative error message.
Beyond the Basics: Exploring Advanced Concepts
As you delve deeper into Python, you’ll encounter more advanced numeric concepts:
- NumPy: A powerful library for numerical computing, offering efficient data structures and functions for complex calculations.
- Pandas: A library for data analysis, providing tools for working with large datasets involving numeric values.
These libraries open doors to exciting possibilities in data science, machine learning, and scientific computing.
Conclusion
By mastering numbers and operations in Python, you’ve laid the groundwork for building exceptional programs. Remember, practice is key! Experiment with different calculations, explore the provided examples, and don’t hesitate to seek help from online communities or tutorials. As you become more comfortable, explore advanced libraries like NumPy and Pandas to unlock the true potential of numeric manipulation in Python. Happy coding!
Read More Posts Like This…
- How Do Websites Work: Basics of Front-End Web Development
- How The Internet Works
- Nutrient Rich Foods for Enhancing Cognitive Function
- Healthy Eating Plans for Different Calorie Goals
FAQs
1. What are some common mistakes beginners make when working with numbers in Python?
- Forgetting to enclose user input in
int()
orfloat()
for conversion. - Using incorrect operators for the desired calculation (e.g.,
//
for integer division instead of/
for floating-point division). - Not handling potential errors like invalid user input.
2. How can I improve my skills in working with numbers in Python?
- Practice writing code to solve various mathematical problems.
- Explore online coding challenges and exercises focused on numeric manipulation.
- Look into Python libraries like NumPy and Pandas to broaden your skillset.
3. What are some real-world applications of numbers and operations in Python?
- Financial calculations and data analysis.
- Scientific computing and simulations.
- Machine learning algorithms that rely on numerical data.
- Game development for physics and character movement.
4. Where can I find more resources to learn about numbers in Python?
- The official Python documentation provides detailed explanations of numeric data types and operators
Practice Quiz
- What data type would you use to store the number of apples you have (3)?
- a)
string
- b)
int
- c)
float
- d)
bool
- a)
- How do you assign the value 10.5 to a variable named
pi
?- a)
pi = int(10.5)
- b)
pi = float("10.5")
- c)
pi = "10.5"
- d)
pi = 10.5
- a)
- What is the result of the expression
5 + 3 * 2
?- a)
13
- b)
16
- c)
10
- d)
6
- a)
- What operator checks if two numbers are equal?
- a)
=
(Assignment) - b)
==
- c)
!=
- d)
<
- a)
- Write Python code to calculate the area of a rectangle with length 7 and width 4.
- What is the output of the following code?
age = 20; print(age > 18)
- a)
True
- b)
False
- c) An error (semicolon not needed after variable assignment)
- d)
age
- a)
- How do you write a Python comment to explain what a line of code does?
- a)
// This is a comment
(This syntax is for C++) - b)
# This is a comment
- c)
/* This is a comment */
(This syntax is for multi-line comments in some languages) - d)
comment = "This is a comment"
- a)
- What does the modulo operator (
%
) do?- a) Multiplication
- b) Division
- c) Exponentiation
- d) Subtraction
- Write Python code to find the remainder when dividing 17 by 5.
- What is the difference between the
and
andor
logical operators?- a)
and
returns True only if both conditions are True, whileor
returns True if at least one condition is True. - b) There is no difference.
- c)
and
checks for equality, whileor
checks for difference. - d)
and
is used for multiplication,or
is used for addition.
- a)
- Complete the following code to check if a number is even:
if number % 2 == ...:
- a)
0
- b)
1
- c)
True
- d)
False
- a)
- Write Python code to ask the user for their age and print a message if they are eligible to vote (18 or older).
- Hint:
age = int(input("Enter your age: ")) if age....
(now complete it)
- Hint:
- What is a loop in Python?
- a) A block of code that repeats until a condition is met.
- b) A way to define functions.
- c) A method for printing text.
- d) A variable that stores multiple values.
- Which loop type iterates a specific number of times based on a counter variable?
- a)
while
loop - b)
for
loop - c)
if
statement - d)
else
statement
- a)
- Write a Python code snippet using a
for
loop to print the numbers from 1 to 5.- Hint: use for loop and range()
- What built-in function can you use to calculate the absolute value of a number (distance from zero)?
- Imagine you have a list of temperatures in Celsius. Write Python code to convert all temperatures in the list to Fahrenheit. (Hint: You’ll need a loop to iterate through the list and a formula to convert Celsius to Fahrenheit).
- What is an error in Python?
- How can you handle errors in your code to prevent it from crashing? (Hint: Look into
try
andexcept
blocks).