Mastering Lists in Python
Imagine you’re a party host, juggling a guest list. You jot down names on a piece of paper, a basic but functional approach. But what if your guest list keeps growing? Soon, keeping track becomes a mess. This is where Python lists come in—your organized toolbox for managing collections of items in your code.
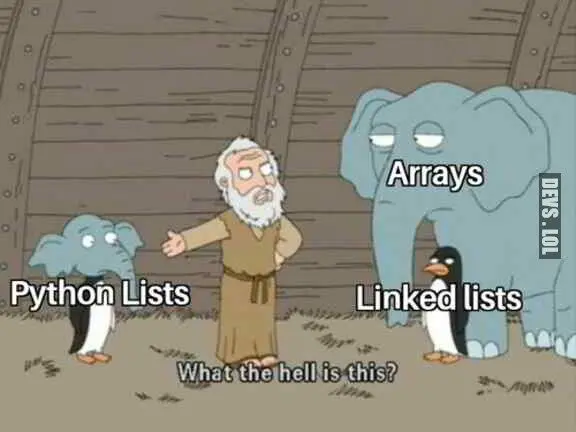
Python lists are versatile, ordered sequences that can store any data type – numbers, strings, even other lists! They’re like flexible containers that adapt to your needs, making them a fundamental building block in Python programming.
How to Initialize Lists in Python
Creating Your Guest List. Let’s get your party started by creating a guest list using lists. Here’s the basic syntax:
guest_list = ["Alice", "Bob", "Charlie"]
This code creates a list named guest_list
containing three string elements: “Alice”, “Bob”, and “Charlie”. But wait, what if your cool neighbor, David, decides to join the party last minute? let us add a new guest to the list.
Adding New Guests, Using the Append() Method
Python offers ways to add elements to your list dynamically. Here’s how to welcome David to the party:
Read More…
- Numbers and Operations In Python Programming
- Introduction to Variables and Data Types in Python
- Unaware Alcohol, Linked to Breast Cancer In Women – WHO
- Nutrient Rich Food for Promoting Weight Management
guest_list.append("David")

The code will output the value shown above. when you add print(guest_list) as the next line of the code to the above Python syntax. The .append()
method adds “David” to the end of the guest_list
, in the terminal section. Now, your list reflects the updated guest count.
Accessing Elements in Lists
Supposing you want to know who’s RSVPed? So, you need to check if a particular guest, like Bob, is on the list. Python allows you to access elements by their position (index) within the list, starting from 0. Here’s how to find Bob:
first_guest = guest_list[0] #"Alice" (since index 0 is the first element)
is_bob_coming = "Bob" in guest_list #Checks if "Bob" is present in the list
print(f"The first guest on the list is: {first_guest}")
print(f"Is Bob attending? {is_bob_coming}") #This should utput: True
Iterating Through Your Guest List with Loops
Iterating using For Loops() Method
Imagine you need to send personalized invitations. Iterating through your list using a for
loop can do this task for you.
for guest in guest_list:
print(f"Sending invitation to: {guest}")
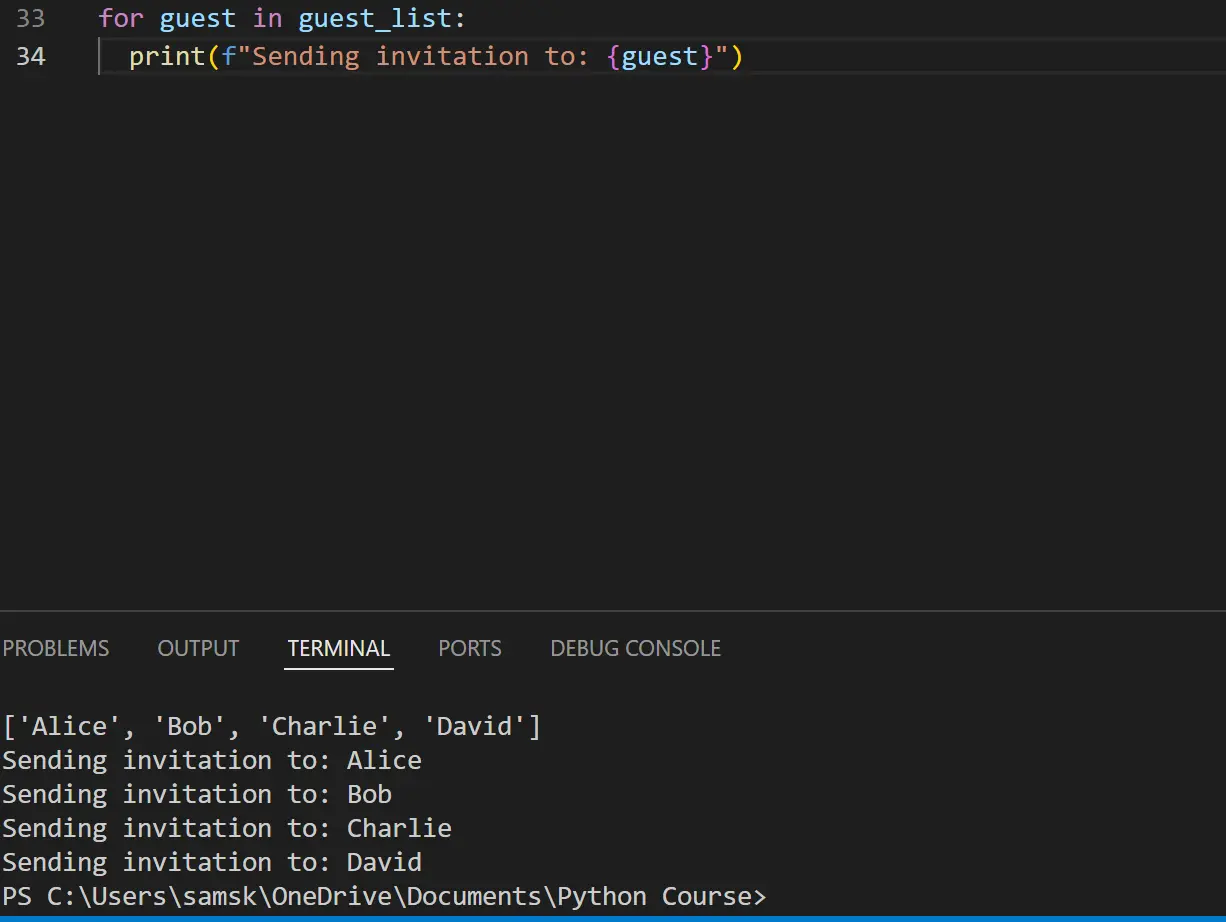
This loop iterates over each guest (represented by the variable guest
) in the guest_list
, printing a personalized invitation message. More example is written below.
magicians = ['alice', 'david', 'carolina']
for magician in magicians:
print(f"{magician.title()}, that was a great trick!")
print(f"I can't wait to see your next trick, {magician.title()}.\n")

The code used the print format print(f” “) to include the element title() methods so that the names of the magicians can start out in a capital letter.
Making a Numerical List using Range() Functions
Sets of numbers are best stored as lists, and Python offers many methods to make working with lists of numbers easier. Your code will continue to function properly even if your lists have millions of items in them if you know how to use these features efficiently.
for value in range(1, 5):
print(value)

The range() function prints only the digits 1 through 4 in this example (Although this code looks like it should print the numbers from 1 to 5, it doesn’t print the number 5). This is a further outcome of programming languages’ frequent off-by-one behavior. When you use the range() function, Python begins counting at the first value you enter and ends when it reaches the second value. The output never includes the end value, which in this case would have been 5, because it stops at that second value. Use range(1, 6) to print the integers from 1 to 5:
Using range() to Make a List of Numbers
Run the below Python script and see the results displayed as a list containing numbers as its elements.
numbers = list(range(1, 6))
print(numbers)
The list() function can be used to directly transform the range() results into a list if you wish to create a list of numbers. A list of numbers is the result of wrapping a call to the range() function with list(). In the above section’s example, we merely printed a string of numbers. The same collection of numbers can be turned into a list using list().
even_numbers = list(range(2, 11, 2))
print(even_numbers)
Additionally, we may instruct Python to skip numbers within a certain range by using the range() function. Python utilizes the value of the third argument you supply to range() as the step size when producing numbers. Above is an example of listing the even numbers between 1 and 10.

In this example, the range() function starts with the value 2 and then adds 2 to that value. It adds 2 repeatedly until it reaches or passes the end value, 11, and produces this result. This is shown on the terminal below in the VSCode Editor.
Almost any set of integers can be generated with the range() method. Think about creating a list of the first ten square numbers, or the square of every integer from 1 to 10, as an example. Two asterisks (**) in Python stand for exponents. You might create a list with the first ten square numbers as follows:
squares = []
for value in range(1, 11):
square = value ** 2
squares.append(square)
print(squares)
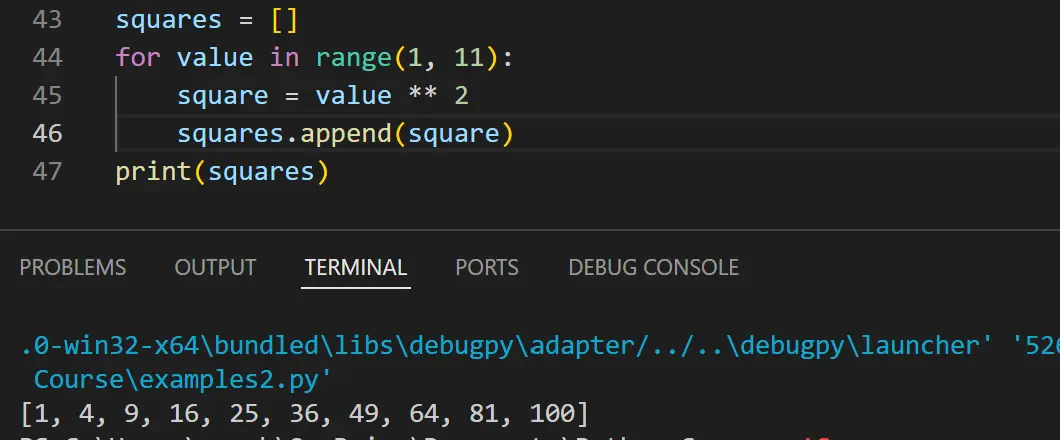
The first line of code in squares is an empty list. At the second, we instruct Python to use the range() method to loop through each integer between 1 and 10. The current value is allocated to the variable square inside the loop after being raised to the second power. The list of squares is updated with each new square value. The list of squares is printed at the end of the loop’s execution. This approach can be modified again when working with lists in Python programming. However, I will leave you figure that out.
Techniques for Deleting from Lists
Oops! It turns out Charlie can’t make it. Let’s remove him from the list using the remove()
method:
guest_list.remove("Charlie")
print(guest_list) # Output: ["Alice", "Bob", "David"]
The .remove() method removes the first occurrence of the specified element (“Charlie”) from the list.
Slicing and Dicing Your List
Imagine you only want to invite the first two guests (Alice and Bob) for a pre-party. Slicing allows you to extract a portion of the list:
guest_list = ['alice', 'bob', 'mariam', 'david']
invite_list = [0:2]
print(invite_list)
#this will output ['alice', 'bob']
Example 1: Basic Slicing
# Original list
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry', 'fig', 'grape']
# Slicing to get the first three elements
first_three = fruits[0:3]
print(first_three)
#Should Output: ['apple', 'banana', 'cherry']
# Slicing to get the last three elements
last_three = fruits[-3:]
print(last_three)
#should Output: ['elderberry', 'fig', 'grape']
The example above is just how you can create more lists from an original list. we can proceed further to make manipulations with lists.
Example 2: Step Slicing
numbers = list(range(1, 11))
#This will give output of [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Slicing with a step of 2
every_other = numbers[::2]
print(every_other)
#This will Output: [1, 3, 5, 7, 9]
# Slicing with a negative step (reversing the list) that is from the last item which is 10
reversed_list = numbers[::-1]
print(reversed_list)
#This will Output: [10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
The method above is called step-slicing. It makes use of an addition argument, a third argument to step size the generating numbers in the list.
Example 3: Slicing with Negative Indices
# Original list
colors = ['red', 'orange', 'yellow', 'green', 'blue', 'indigo', 'violet']
# Slicing to get the elements from 'yellow' to 'blue'
middle_slice = colors[2:5]
print(middle_slice) # Output: ['yellow', 'green', 'blue']
# Slicing to get the elements from 'blue' to 'orange' (negative indices)
reverse_middle_slice = colors[-3:-6:-1]
print(reverse_middle_slice) # Output: ['blue', 'green', 'yellow']
Example 4: Slicing Without Start or End Indices
# Original list
letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g']
# Slicing to get all elements (copy of the list)
all_elements = letters[:]
print(all_elements) # Output: ['a', 'b', 'c', 'd', 'e', 'f', 'g']
# Slicing to get elements from the third element to the end
from_third = letters[2:]
print(from_third) # Output: ['c', 'd', 'e', 'f', 'g']
# Slicing to get elements from the beginning to the fourth element
to_fourth = letters[:4]
print(to_fourth) # Output: ['a', 'b', 'c', 'd']