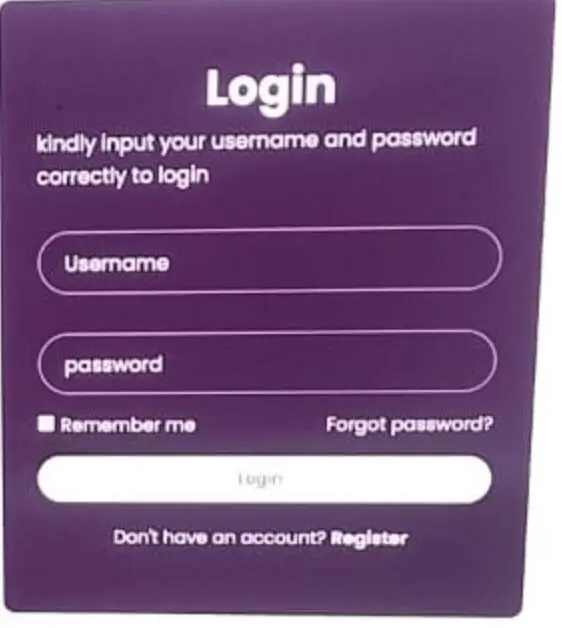
Programming Exercise 1
In today’s session, we will try practicing some programming exercises in Python programming. We will take some practice questions; we will write a python code, by creating a list of 5 people called names. then ask the user to input their name. We will save the name in the list. Then as the user to input a password, save the password in another list called password. Then request the user to state their name, check in the list if their name is the list camed names. If it is, we will print, user found. Then ask them for their password, if it is there, tell them it is found and print access granted. If the person’s password is 12345, tell the person that the password is for a stupid person.
# Create a list of 5 people called names
names = ["Alice", "Bob", "Charlie", "David", "Eve"]
passwords = []
# Ask the user to input their name and save it in the list
user_name = input("Enter your name: ")
names.append(user_name)
# Ask the user to input a password and save it in the passwords list
user_password = input("Enter your password: ")
passwords.append(user_password)
# Request the user to state their name
check_name = input("State your name: ")
# Check if the name is in the list
if check_name in names:
print("User found.")
# Ask for the password
check_password = input("Enter your password: ")
# Check if the password is correct
if check_password in passwords:
if check_password == "12345":
print("Password is for a stupid person.")
else:
print("Access granted.")
else:
print("Incorrect password.")
else:
print("User not found.")
The above provided Python code simulates a basic user authentication system. It creates lists to store names and passwords, allows a user to register, and then checks their credentials for login.
The Result

We can also write the python program code as:
#create a list called names
names = ["Alice", "Bob", "Charlie", "David", "Eve"]
#create an empty list to contain the passwords
passwords = []
#ask users for their name and append it to the names
names.append(input('what is your name?'))
#ask user for their password and put in the password
passwords.append(input('And what is your password?'))
#now, check their password
checkUserName = input("What is your name?")
#use a conditional statement to check for the names and the password
if checkUserName in names:
print(f'Welcome {checkUserName}, your name is found on the list')
print('kindly input your password')
checkPassword = input('please verify your password by inputting your password')
if checkPassword in passwords:
print(f"Well done {checkUserName}, password correct!")
elif checkPassword == "1234":
print("Wrong!, That is a stupid passsword")
else:
print("Wrong password")
else:
print(f'{checkUserName} not found on the list')
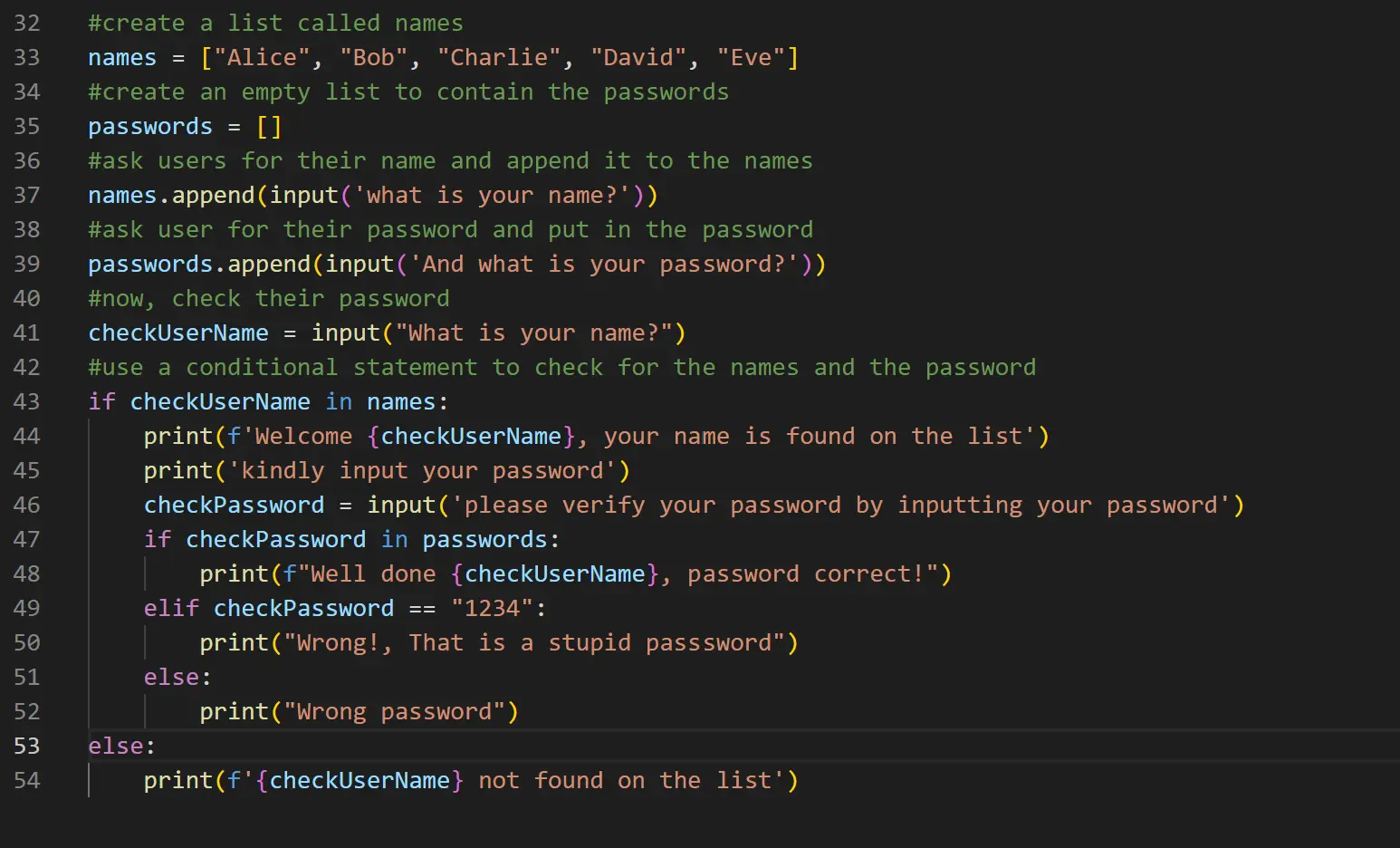
The Result
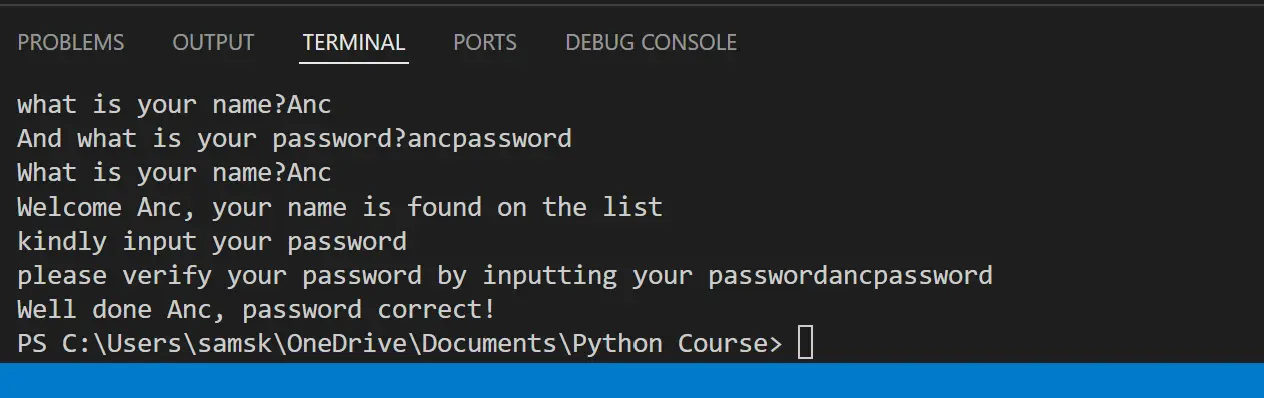
Notice that we didn’t follow the instructions to the latter. It said that it should print “Access Granted” when the user has passed all the checks. However, ours is printing welcome. TO fix this, we can just go and modify the print syntax to do this.
Lastly, the user inputs are on the same line as the text commands or inquiries themselves. Let us try and fix this. We will try and add a next line or new line syntax to the string text in the input commands.
#create a list called names
names = ["Alice", "Bob", "Charlie", "David", "Eve"]
#create an empty list to contain the passwords
passwords = []
#ask users for their name and append it to the names
names.append(input('what is your name?\n'))
#ask user for their password and put in the password
passwords.append(input('And what is your password?\n'))
#now, check their password
checkUserName = input("Please kindly state your name?")
#use a conditional statement to check for the names and the password
if checkUserName in names:
print(f'Welcome {checkUserName.title()}, your name is found on the list')
checkPassword = input('please verify your password by inputting your password\n')
if checkPassword in passwords:
print(f"Well done {checkUserName.title()}, password correct, Access granted!")
elif checkPassword == "1234":
print("Wrong!, That is a stupid passsword")
else:
print("Wrong password")
else:
print(f'{checkUserName} not found on the list')
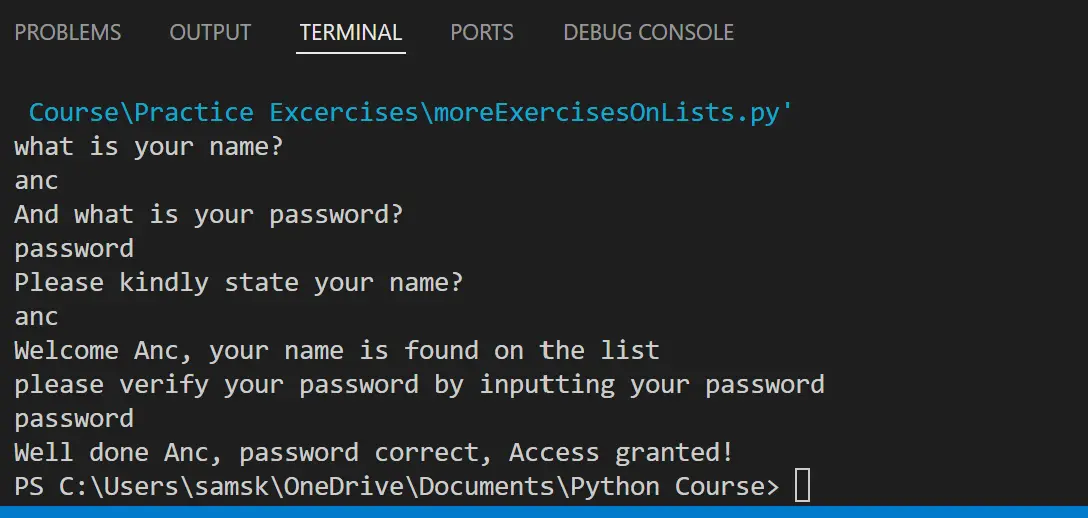
Read Also…
Programming Exercise 2
We will write a python code, by creating a list of that can contain ONLY 5 people called names. Then ask the user to input their name. We will save the name in the list. Then as the user to input a password, save the password in another list called password. Then request the user to state their name, check in the list if their name is the list named names. If it is, we will print, user found. Then ask them for their password, if it is there, tell them it is found and print access granted. If the person’s password is 12345 or password, tell the person that the password is for a stupid person.