When writing programs in Python programming language, you can decide that your program shouldn’t execute from the beginning of your line of code. You have the option to run one of multiple instructions, skip instructions, or repeat instructions. Actually, you virtually may never want your programs to run from the very first line of code and simply go through each and every line until they finish. Depending on the situation, flow control statements can determine which Python instructions to run.

The above image shows a flowchart for what to do if it’s raining. If you follow the path made by the arrows from Start to End, you can start seeing this as an algorithm and hence, convert them to Python codes. These flow control statements directly correspond to the symbols in a flowchart.
Boolean Values with Flow Control
You must first understand how to express those branching points as Python code and how to represent those yes/no options before you can learn about flow control statements. Let’s examine Boolean values, comparison operators, and Boolean operators in order to achieve that.
The Boolean data type has two possible values: True and False, whereas the integer, floating-point, and string data types can have an infinite number of possibilities. (This data type is named after the famous mathematician George Boole, so Boolean is capitalized.) The Boolean values True and False do not have quotations around strings when input as Python code; instead, they always begin with a capital T or F and end with lowercase letters.

type Python in the search field of your PC to open the interactive shell, enter the following as above. (A few of these instructions are purposefully wrong; if you follow them, error messages will display.)

However, when we type true with only the small letter “t”, we get error as shown in the image above.
Comparison Operators
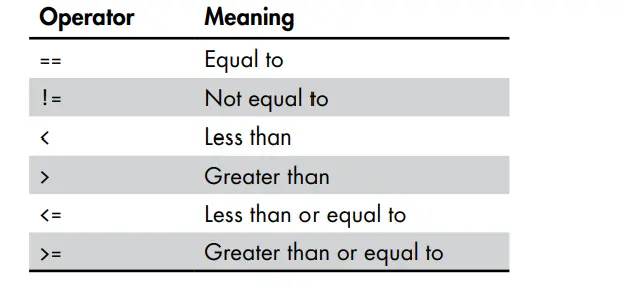
These are known for comparing two values and evaluating to a single Boolean value. Hence, they are called comparison operators, also known as relational operators. Depending on the values you provide, these operators evaluate to True or False. Now let’s test a few operators, beginning with == and!=.
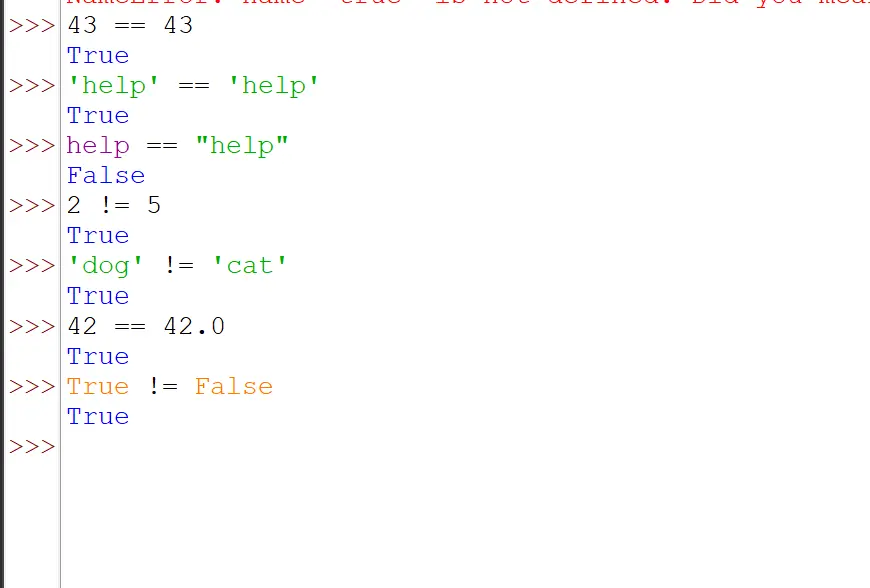
Know the Difference Between == and = operators
It is possible that you have observed that the = operator (assignment) has a single equal sign, whereas the == (equal to) operator has two equal signs. These two operators are easy to get confused for one another. Just keep the following in mind:
- The == operator (equal to) inquires as to whether two values are identical.
- However, in the = operator, the value on the right is assigned to the variable on the left by the operator =.
Notice that both the == operator (equal to) and the != operator (not equal to) are made up of two letters. This will help you remember which is which.
Read More…
- Python Lists Comprehension
- Working With Lists In Python
- Nutrient Rich Foods for Enhancing Cognitive Function
- Creative Ways for Students to Earn Money Online in 2024
Flow Control: The Boolean Operators
To compare Boolean values, utilize the three Boolean operators: and, or, and not. They evaluate expressions down to a Boolean value, much like comparison operators do.

The and and or operators always take two Boolean values (or expressions), so they’re considered binary operators. The and operator evaluates an expression to True if both Boolean values are True; otherwise, it evaluates to False.

On the other hand, the or operator evaluates an expression to True if either of the two Boolean values is True. If both are False, it evaluates to False.
Flow Control: The not Operator
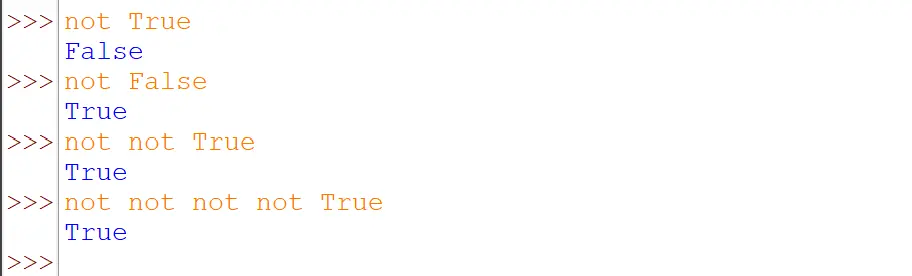
When looking at the not operator, unlike the and and or operators, the not operator operates on only one Boolean value (or expression). This makes it a unary operator. The not operator simply evalulates to the opposite Boolean value.
A Mixture of Boolean and Comparison Operators
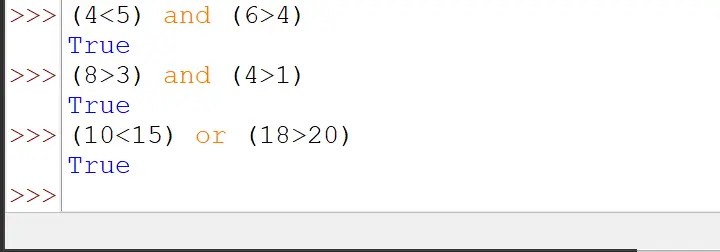
While the expressions like 4 < 5 aren’t Boolean values, they are expressions that evaluate down to Boolean values. In the above expression, the computer will evaluate the expression on the left first, and then it will evaluate the expression on the right. When it knows the Boolean value for each.

In the end, t will evaluate the whole expression down to one Boolean value. You can think of the computer’s evaluation process for (4 < 5) and (5 < 6) as shown above in the image.
Just like the math operators do, the Boolean operators have an order of operations. After any math and comparison operators evaluate, Python evaluates the not operators first, then the and operators, and then the or operators.
The Elements of Flow Control
When dealing with flow control statements, we often start with a part called the condition and this is always followed by a block of code called the clause. It is always important to know what Python’s specific flow control statements are.
Conditions
The Boolean expressions we have written so far could all be considered, which are the same thing as expressions; whereas condition is just a more specific name in the context of flow control statements. This is because conditions always evaluate down to a Boolean value, True or False. A flow control statement decides what to do based on whether its condition is True or False, and almost every flow control statement uses a condition.
Blocks of Code
Often times we group lines of Python code into blocks. A block begins and ends from the indentation of the lines of code. There are three rules for blocks namely:
- Blocks begin when the indentation increases.
- Blocks can contain other blocks.
- Blocks end when the indentation decreases to zero or to a containing block’s indentation.

The first block of code starts at the line print(‘welcome, Miss Anichebe’) and contains all the lines after it. Inside this block is another block, which has only a single line in it: print(‘Access granted.’). The third block is also one line long: print(‘wrong password.’).
Flow Control Statements: If Statements
Let us write a program that checks if a name written is actually Alice. and if the name is Alice, it will print out the word Alice on the terminal.
name = input("what is your name?")
if name == 'Alice':
print('Hi, Alice.')
The flow control statement diagram of the Python code is drawn below as:

Conclusion
Flow control statements are the backbone of Python programming, allowing you to dictate the execution flow of your code. By mastering these statements, you can make decisions, repeat tasks, and handle different situations, making your programs more dynamic and adaptable. From simple conditional statements to complex nested loops, flow control empowers you to build efficient and versatile Python applications. As you continue your programming journey, remember to leverage these powerful tools to create well-structured and logical code.