In this tutorial, we will create Python simple games that are fun and easier to program. We will start very small and move up to complex details that could factor other areas of the games. Let use begin. Imagine a game of users shooting down flying ducklings. When user shoots down a duckling, he/she can show what color of flying duckling he/she shot down and he/she will win a certain point for doing so.
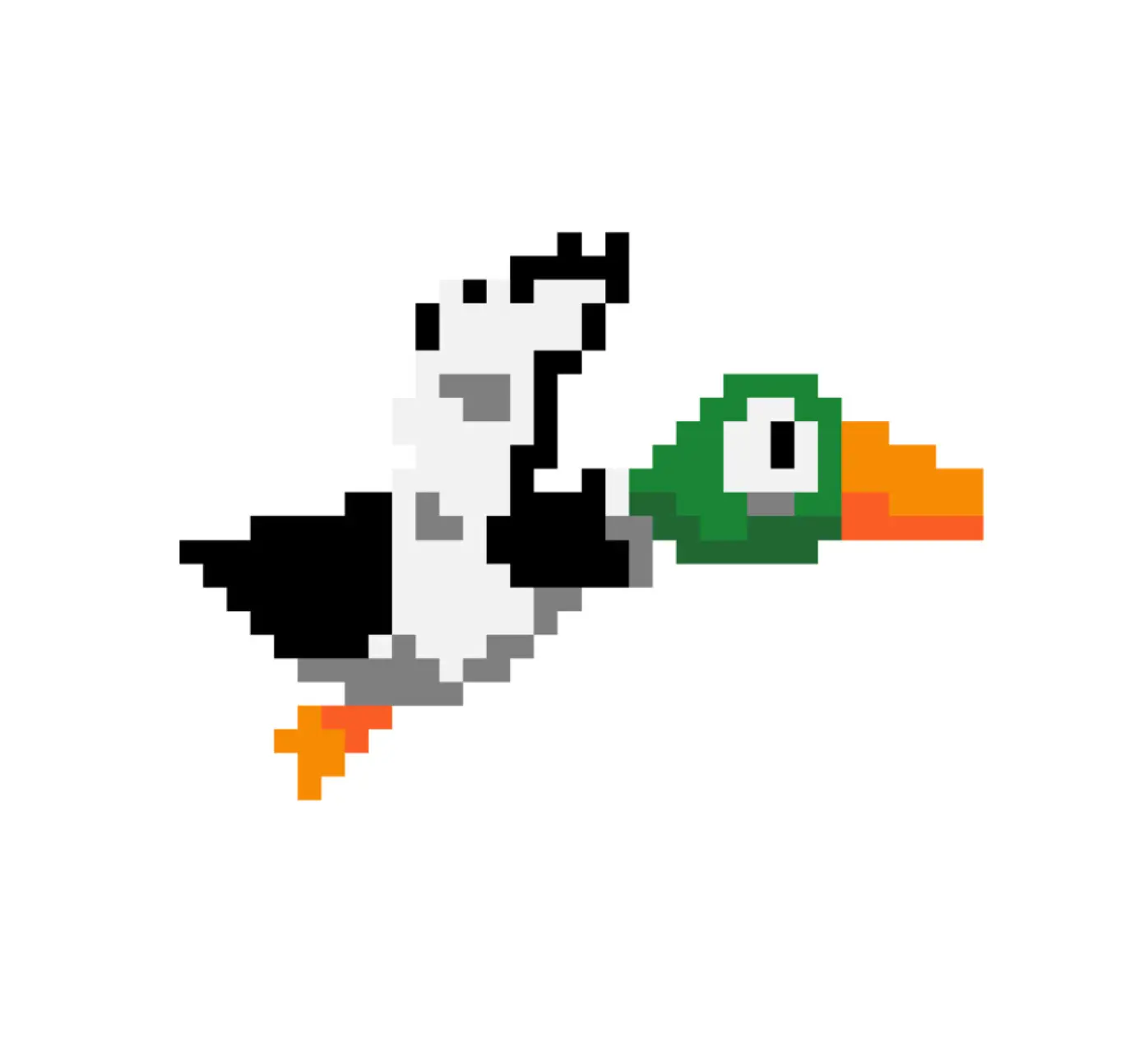
Now, we don’t have to program this game from the actual gaming environment and all advanced python libraries and code. We will use creating of list to input the colors of the flying ducklings. In our list, we will limit the colors of the flying ducklings to be only “red”, “yellow” and “green”.
points = 0 #define the points a user gets for playing
duckColor = ['red', 'yellow', 'green'] #create a list to house the color of the ducklings shot down
#ask the user what color of ducling they shot down
askUser = input("What color of duckling did you shoot down?")
askUser = askUser.lower() #convert the response the lowercase
if askUser == 'red':
points += 5
print(f"\nYou just earned {points} for shooting down a {askUser} color duckling")
elif askUser == 'yellow':
points += 10
print(f"\nYou just earned {points} for shooting down a {askUser} color duckling")
elif askUser == 'green':
points += 20
print(f"\nYou just earned {points} for shooting down a {askUser} color duckling")
print("Thank you for playing")
Since we only have three (3) colors for these flying ducklings, we have used if and elif conditional statements to assign what points a user gets when he/she shoots down a certain flying duckling color. And we used the user input function to ask the user what color of duckling they shot down. And in the end, we will thank them for playing the game.
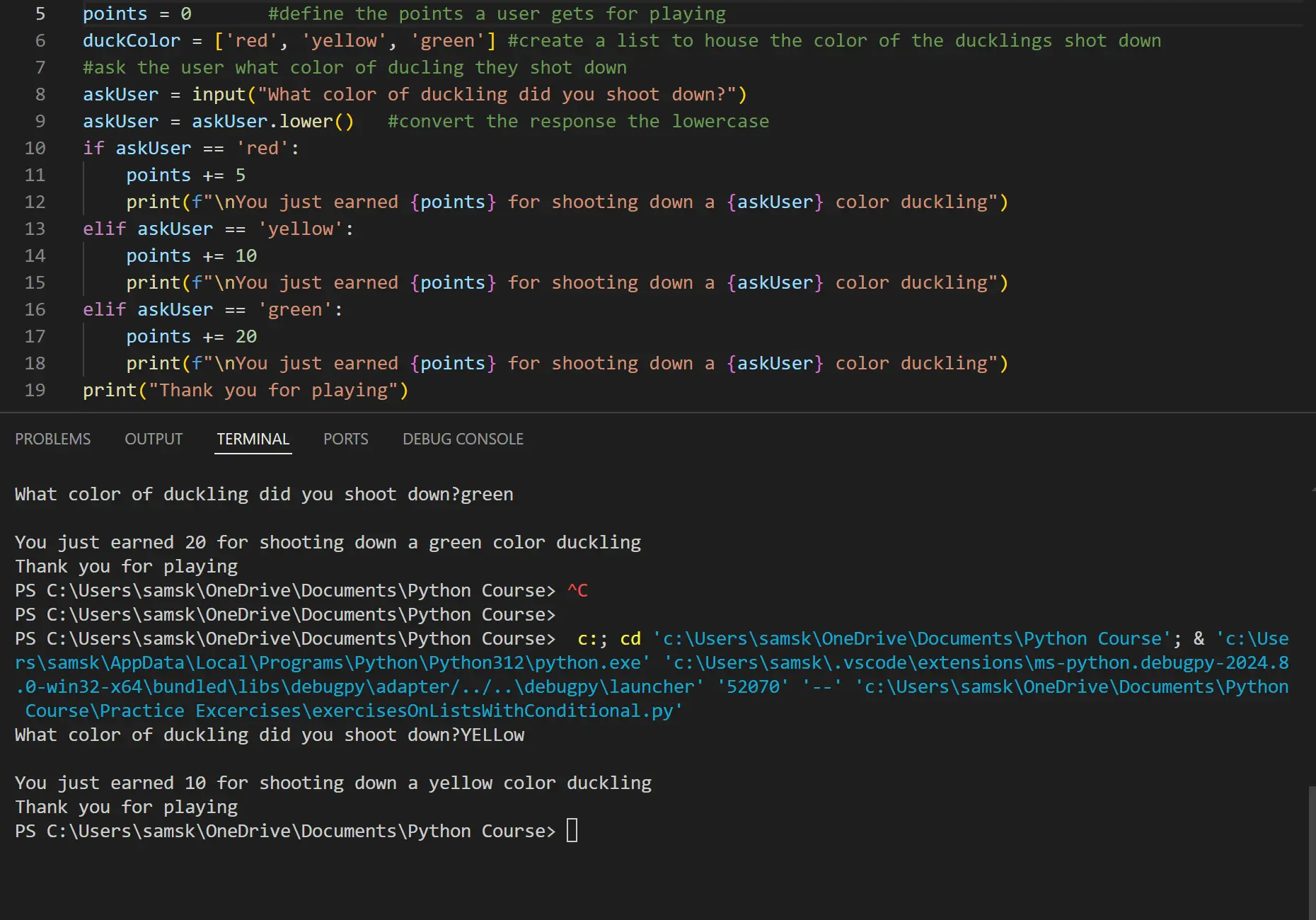
We have already specified the initial points to be zero in code line 5. and we used the lower() method to convert their response to lowercase strings. Then do the comparism. The result is shown in the image above.
Now, let us imagine user makes a mistake when typing his/her response. Instead of typing out “red” or “yellow” or “green”, he/she types in a different word, we can use an else statement to take care of this. We modify our python code as:
points = 0 #define the points a user gets for playing
duckColor = ['red', 'yellow', 'green'] #create a list to house the color of the ducklings shot down
#ask the user what color of ducling they shot down
askUser = input("What color of duckling did you shoot down?")
askUser = askUser.lower() #convert the response the lowercase
if askUser == 'red':
points += 5
print(f"\nYou just earned {points} for shooting down a {askUser} color duckling")
elif askUser == 'yellow':
points += 10
print(f"\nYou just earned {points} for shooting down a {askUser} color duckling")
elif askUser == 'green':
points += 20
print(f"\nYou just earned {points} for shooting down a {askUser} color duckling")
else:
print(f"\nSorry the color {askUser} isn't recognized. Please pick the game's recommended flying duckling color")
print("Thank you for playing")
We tell the user that the color he/she typed in isn’t recognized using the else statement. The result is shown in the picture below.
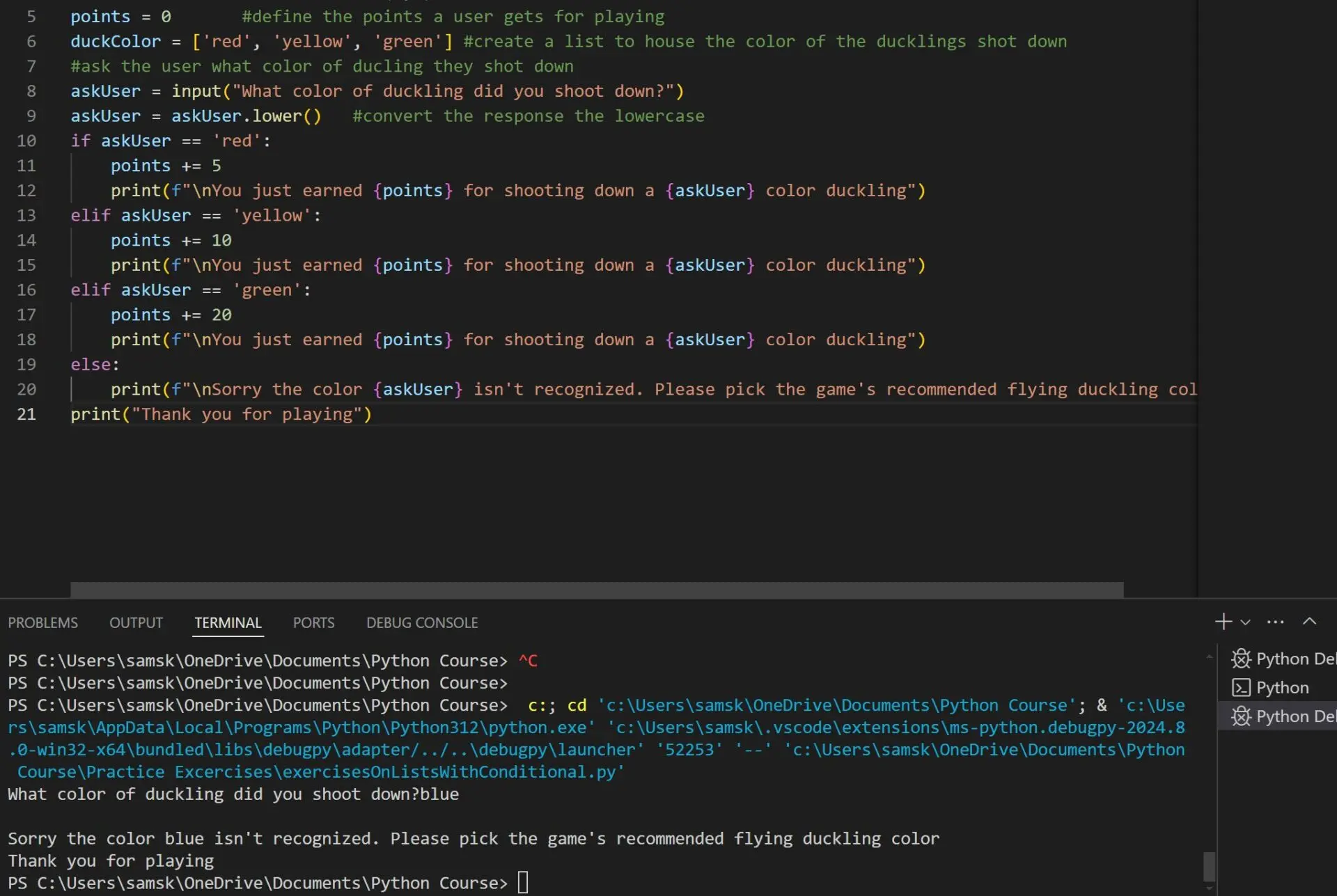
What if the user wants to keep playing after making his/her mistakes? Well then, we can add a while loop or a for loop to keep the user playing. Let us look at the cases:
Using a While Loop in this Python Simple Game
points = 0
duckColor = ['red', 'yellow', 'green']
while True:
askUser = input("What color of duckling did you shoot down? (Type 'quit' to exit): ")
askUser = askUser.lower()
if askUser == 'quit':
break
elif askUser in duckColor:
if askUser == 'red':
points += 5
elif askUser == 'yellow':
points += 10
elif askUser == 'green':
points += 20
print(f"\nYou just earned {points} points for shooting down a {askUser} color duckling")
else:
print(f"\nSorry, the color {askUser} isn't recognized. Please pick a valid flying duckling color.")
print("Thank you for playing!")
Read Python Programming Exercises | Practice Questions
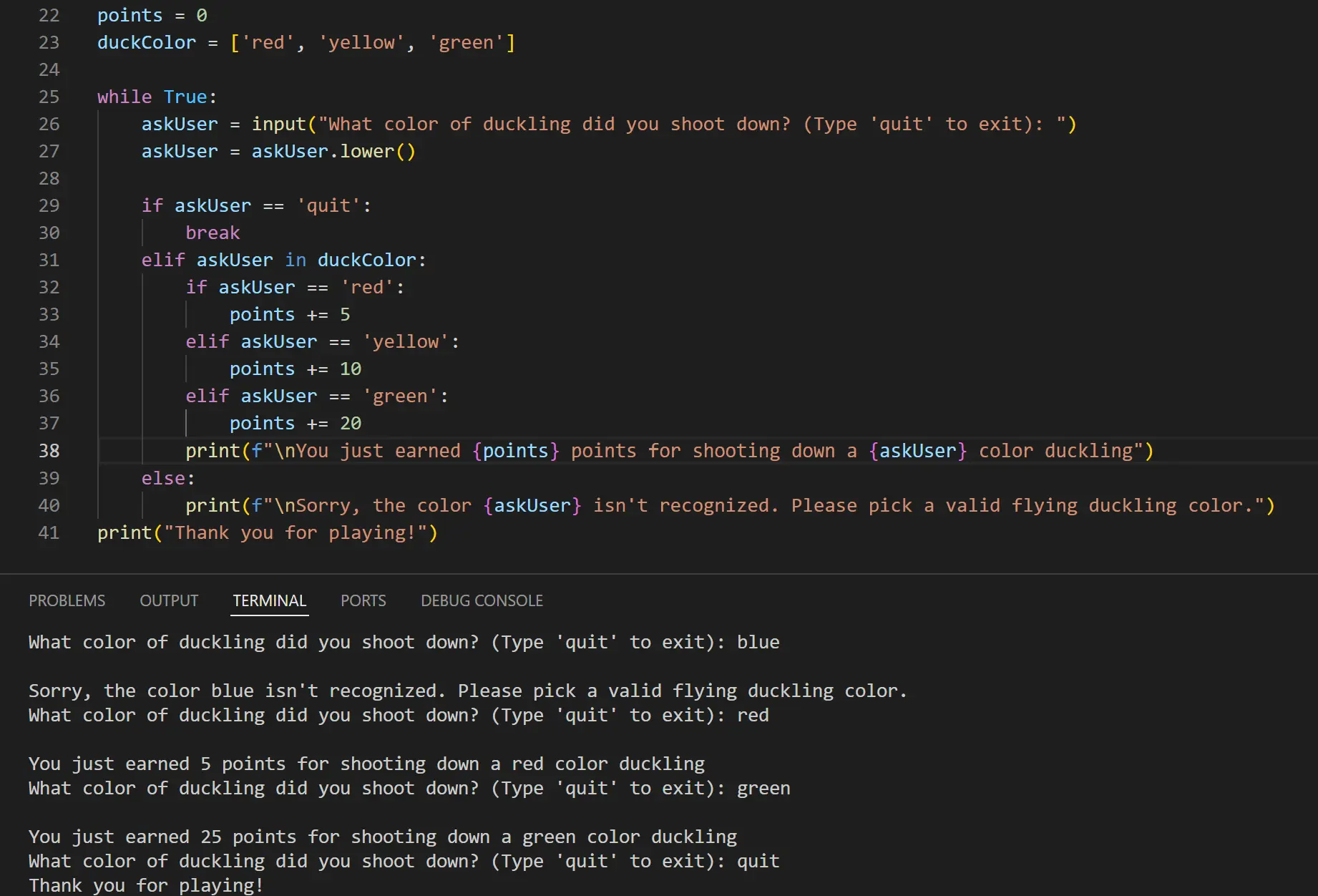
The python code above uses the while loop to repeat the input to the user even if they made a mistake. Note that we have converted the user’s input to a lower case string, so even if they typed their response in different case letters, it will still recognize it. We used the break syntax to exit the while loop, and this would kick in when the user types the word “quit”.
The result of this is shown in the terminal and to test for the repetition, I have entered a wrong input and the code returned to me that color isn’t recognized. And when the right color is types, it produces the right points.
Now, let us redo the whole thing using a for loop. To rewrite the given code using a for loop, you’ll need to decide on the number of iterations the loop should execute, as a for loop in Python iterates over a sequence or range. Since the original code uses a while True loop that only exits when the user types ‘quit’, one approach is to use a sufficiently large range for the for loop. However, it’s more common and practical to use the while loop for this type of problem. Here’s an example where we ask the user for the number of times they want to play before starting the loop.
points = 0
duckColor = ['red', 'yellow', 'green']
num_tries = int(input("How many times do you want to play? "))
for _ in range(num_tries):
askUser = input("What color of duckling did you shoot down? (Type 'quit' to exit): ")
askUser = askUser.lower()
if askUser == 'quit':
break
elif askUser in duckColor:
if askUser == 'red':
points += 5
elif askUser == 'yellow':
points += 10
elif askUser == 'green':
points += 20
print(f"\nYou just earned {points} points for shooting down a {askUser} color duckling")
else:
print(f"\nSorry, the color {askUser} isn't recognized. Please pick a valid flying duckling color.")
print("Thank you for playing!")
In this version, the number of iterations is defined by the num_tries
variable, which the user sets at the beginning. The loop will run that many times unless the user types ‘quit’ to exit early. In the code above, the total code adds up the total points earned by the player. And when the player types ‘quit’, it will exit the for loop.
Let us look at when the player or user keeps picking up the high-point colors. This can be considered as cheating; supposedly, the user keeps typing the color ‘green’ to gain 20 points each time. To try and solve this, and ensure transparent user input, we can have the code randomly generate the color code, and ask the user to type the color code they see.
points = 0
duckColor = ['red', 'yellow', 'green']
while True:
#generate a random number between 1 and 3
randomNumber = random.randint(1,3)
if randomNumber == 1:
color = 'red'
if randomNumber == 2:
color = 'yellow'
if randomNumber == 3:
color = 'green'
print(color)
askUser = input("What color of duckling did you shoot down? (Type 'quit' to exit): ")
askUser = askUser.lower()
if askUser == 'quit':
break
elif askUser == color:
if askUser == 'red':
points += 5
elif askUser == 'yellow':
points += 10
elif askUser == 'green':
points += 20
print(f"\nYou just earned {points} points for shooting down a {askUser} color duckling")
else:
print(f"\nSorry, the color {askUser} isn't recognized. Please pick a valid flying duckling color.")
print(f"Your earned a total {points}\nThank you for playing!")
Conclusion
The simple exercise carried out above is all about practicing how to use conditional statements in python programming with a combination of while loops and for loops to create some simple interesting games for beginners. You can as well improve on it and make it more better and fun! Let me know in the comments.