Here are 30 Python challenge exercises covering the topics we have done so far:
Operators and Expressions
- Write a program to calculate the area of a triangle given its base and height using multiplication and division operators.
- Write a program that swaps the values of two variables without using a temporary variable.
- Evaluate the expression
((10 + 3) * 2 - 4 / 2)
and explain the operator precedence used.
- Write a program to calculate the area of a triangle given its base and height using multiplication and division operators.
#to calculate the area of a triangle
#halve multiply by base multiply by height
base = input("choose a number of your choice?\n")
height = input("choose a second number of your choice?\n")
input_1 = 1 / 2
multiplicationOperator = input_1 * int(base) * int(height)
print(f"The quotient of {base} by {height} by {input_1} is: {multiplicationOperator}\n")
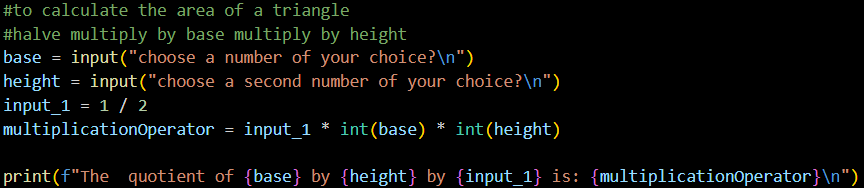
3. Evaluate the expression
((10 + 3) * 2 - 4 / 2)
and explain the operator precedence used.
#using BODMAS
additionOperator = 10 + 3 #solving the bracket first
divisionOperator = 4 / 2 #solving division next after bracket
multiplicationOperator = int(additionOperator) * 2 #solving multiplication after division
subtractionOperator = int(multiplicationOperator) - int(divisionOperator) #solving subtraction last to get the final answer
print(f"The answer is: {subtractionOperator}\n") #print the value

Data Types, String Concatenation, and Replication
- Create a program that asks for the user’s first and last name and prints them together using string concatenation.
- Write a program that replicates a string
n
times, wheren
is a user-provided integer. - Convert a floating-point number to an integer and a string. Print the results.
4. Create a program that asks for the user’s first and last name and prints them together using string concatenation.
name = input("write your first name?\n")
Surname = input("write your surname?\n")
fullname = ("my name is " + Surname.title() + " " + name.title())
print(f"{fullname}\n")
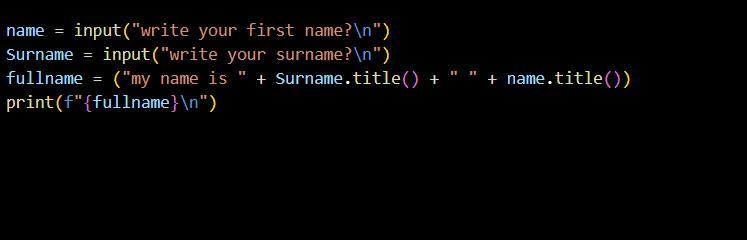
6. Convert a floating-point number to an integer and a string. Print the results.
float_no = float(input("write down a number\n"))
int_no = int(float_no)
string = (int_no)
print(f"converted to string: {int_no}\n")
print(f"The integer number of the float number is: {string}\n")
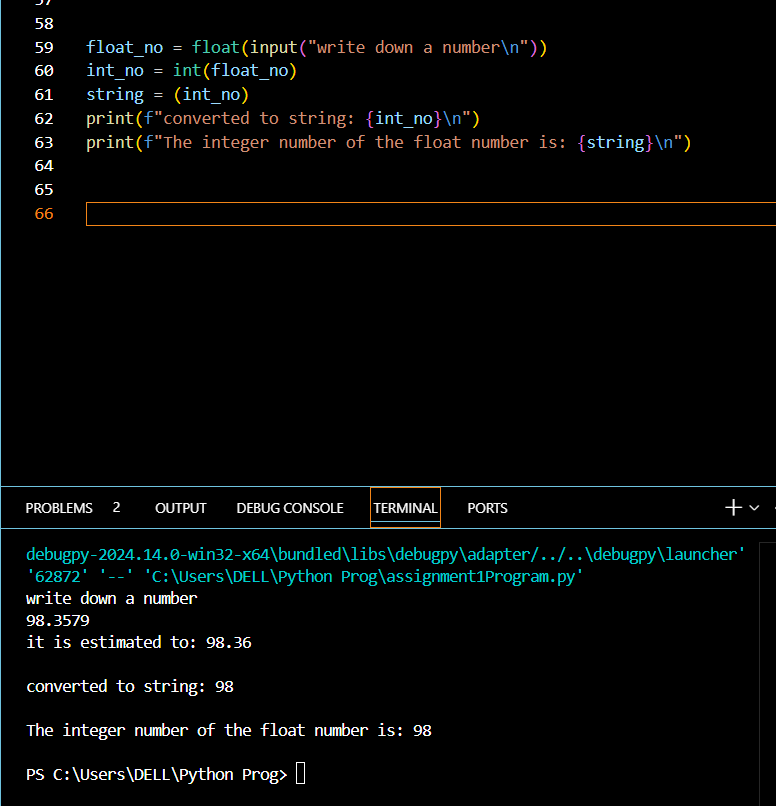
Input and len()
Function
- Write a program that takes a user’s full name as input and prints the number of characters in their name.
- Create a program that asks for the user’s age and calculates the year they were born.
- Use the
len()
function to check the length of a password and display whether it is “Weak”, “Moderate”, or “Strong”.
7. Write a program that takes a user’s full name as input and prints the number of characters in their name.
name = input("write your name?\n") #User input his/her name
fullname = name
Tname = print(len(fullname)) #computer prints the total values of the name eg: akachukwu = 9
print(f"{Tname}\n") #computer prints it in a new line using the dictionary above in line 37

8. Create a program that asks for the user’s age and calculates the year they were born.
age = input("what's your age?\n")
year = 2024
birth = int(year) - int(age)
print(f"the year you were born is: {birth}\n")

Comparison Operators
- Write a program to check if a given number is greater than, less than, or equal to 50.
- Take two inputs from the user and compare if the first input is lexicographically smaller than the second.
- Demonstrate the difference between
==
and=
by writing a small program with both.
10. Write a program to check if a given number is greater than, less than, or equal to 50.
value = input("write a value of your choice?\n") #user is ask to write a value
value_1 = 50 #computers comparing value is 50
greater = int(value) > int(value_1) #it is compared using comparism sign
lesser = int(value) < int(value_1)
if greater:
print(f"{value} is greater") #using conditional statement to compare
elif lesser:
print(f"{value} is lesser")
else:
print(f"{value} is equal")
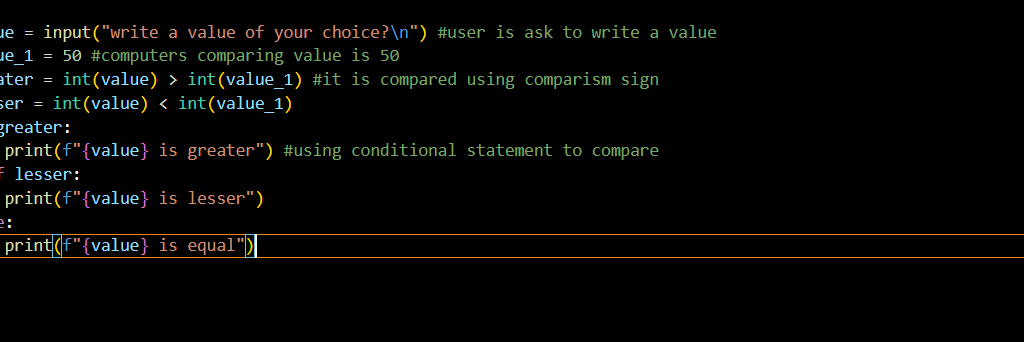
11. Take two inputs from the user and compare if the first input is lexicographically smaller than the second.
input1 = input("Enter the first string\n")
input2 = input("Enter the second string\n")
# Compare the strings lexicographically
if input1 < input2:
print(f"'{input1}' is lexicographically smaller than '{input2}'.")
elif input1 > input2:
print(f"'{input1}' is lexicographically greater than '{input2}'.")
else:
print(f"'{input1}' is lexicographically equal to '{input2}'.")
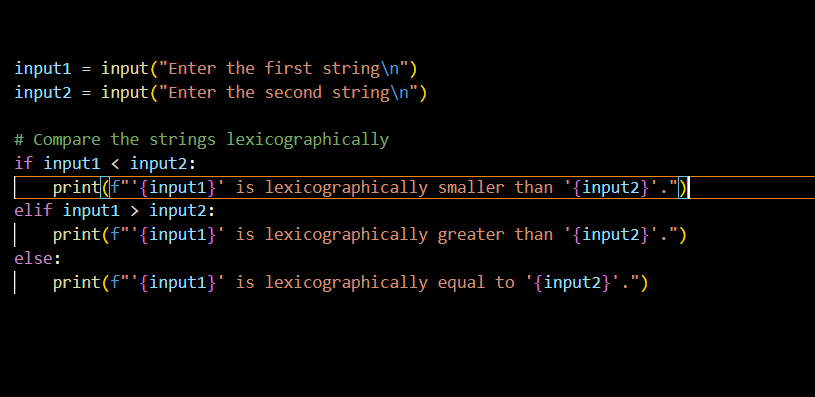
12. Demonstrate the difference between
==
and=
by writing a small program with both.


Boolean Operators
- Write a program to check if a number is divisible by both 3 and 5.
- Take three user inputs and check if all of them are non-negative numbers using boolean operators.
- Combine comparison and boolean operators to check if a number is between 10 and 20 (inclusive).
13. Write a program to check if a number is divisible by both 3 and 5Write a program to check if a number is divisible by both 3 and 5
no = int(input("write a number: "))
modulusOperator = no % 3 == 0
modulusOperator2 = no % 5 == 0
if modulusOperator and modulusOperator2 is True:
print(f"{no} is divisible by 3 and 5")
else:
print(False)
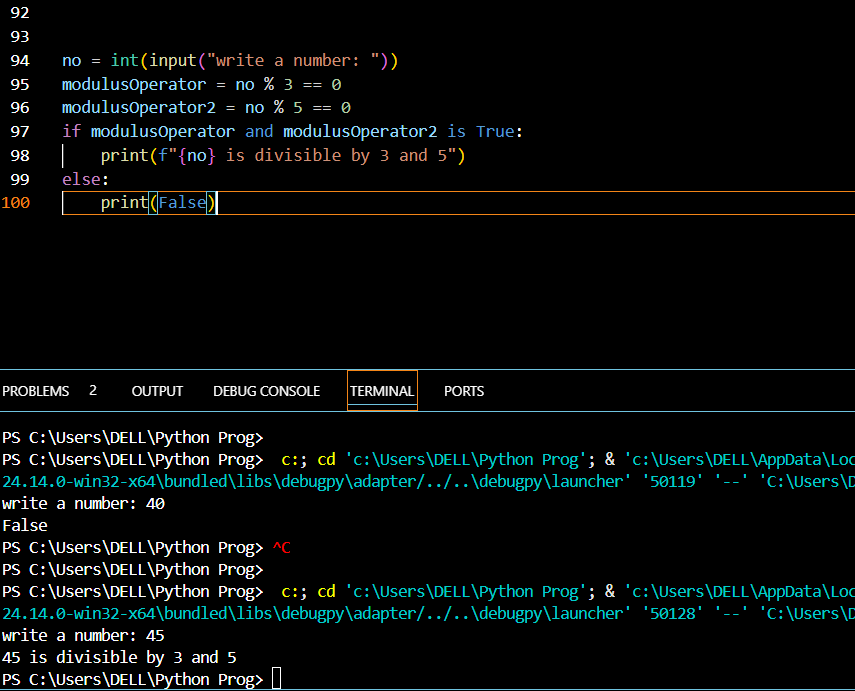
14. Take three user inputs and check if all of them are non-negative numbers using boolean operators.
no1 = float(input("write a number: "))
no2 = float(input("write a second number: "))
no3 = float(input("write a third number: "))
num1 = no1 >= 0
num2 = no2 >= 0
num3 = no3 >= 0
if num1 and num2 and num3 is True:
print("all numbers are non negative")
else:
print("some numbers are negative")
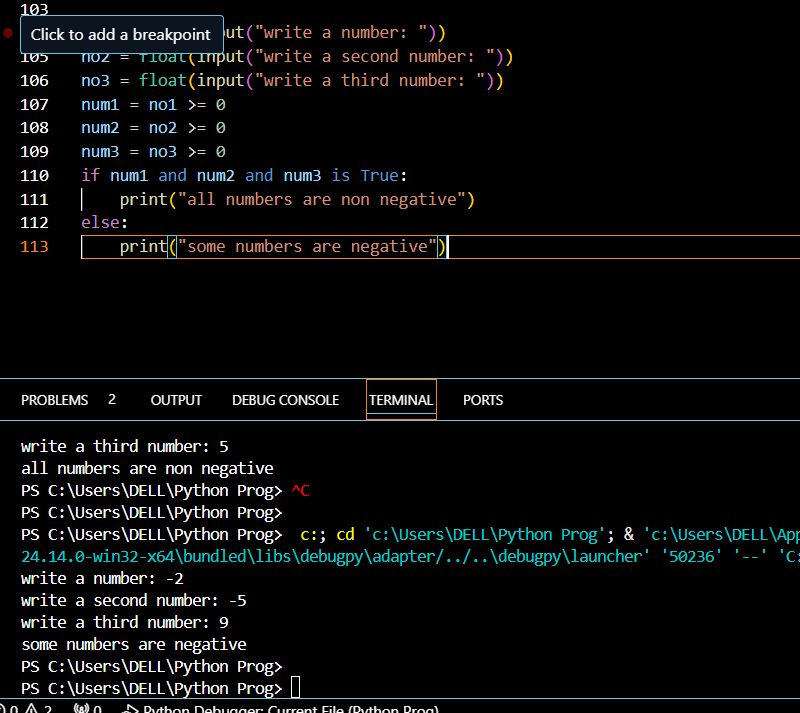
15. Combine comparison and boolean operators to check if a number is between 10 and 20 (inclusive).
no_input = int(input("write a number: "))
if 10 <= no_input <= 20:
print("it is inclusive")
else:
print("it is not inclusive")
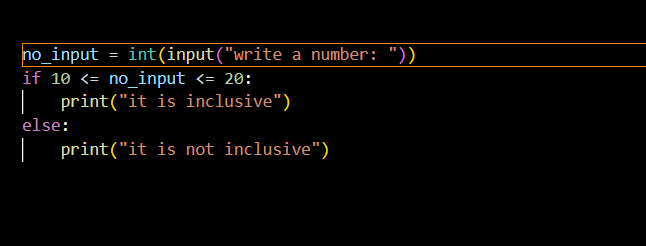
Flow Control and Conditional Statements
- Write a program to display all even numbers between 1 and 50 using a
for
loop. - Create a
while
loop that counts down from 10 to 0 and prints “Liftoff!” at the end. - Write a program that prints all numbers from 1 to 20 but skips multiples of 3 using the
continue
statement. - Use a
for
loop andbreak
to stop iterating through a list of numbers when you find a negative number. - Write a program to calculate the factorial of a user-provided number using a
while
loop.
Combining Concepts
- Ask the user for their age, and based on the input, print whether they are a “Child”, “Teenager”, “Adult”, or “Senior”.
- Write a program that takes a string input and prints “Palindrome” if the string reads the same backward.
- Use a
for
loop to generate a multiplication table for a number input by the user. - Create a program that calculates the sum of all integers between two numbers provided by the user.
- Write a program to find the largest and smallest numbers in a list using a
for
loop.
Bonus Challenges
- Create a program that asks for a user’s full name and reverses the order of their name (e.g., “John Doe” becomes “Doe John”).
- Write a guessing game where the user has to guess a random number between 1 and 100. Use
while
loops to keep asking until they guess correctly. - Create a program to check if a user-input string contains both uppercase and lowercase letters.
- Write a program to count the vowels in a user-provided string.
- Write a program that takes a list of numbers and prints only the prime numbers from the list.